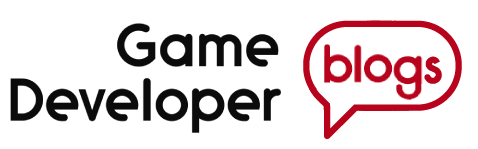
Featured Blog | This community-written post highlights the best of what the game industry has to offer. Read more like it on the Game Developer Blogs or learn how to Submit Your Own Blog Post
Unity + Kongregate: a beginner beginner's guide to badges
A guide to implement Kongregate badges via Unity
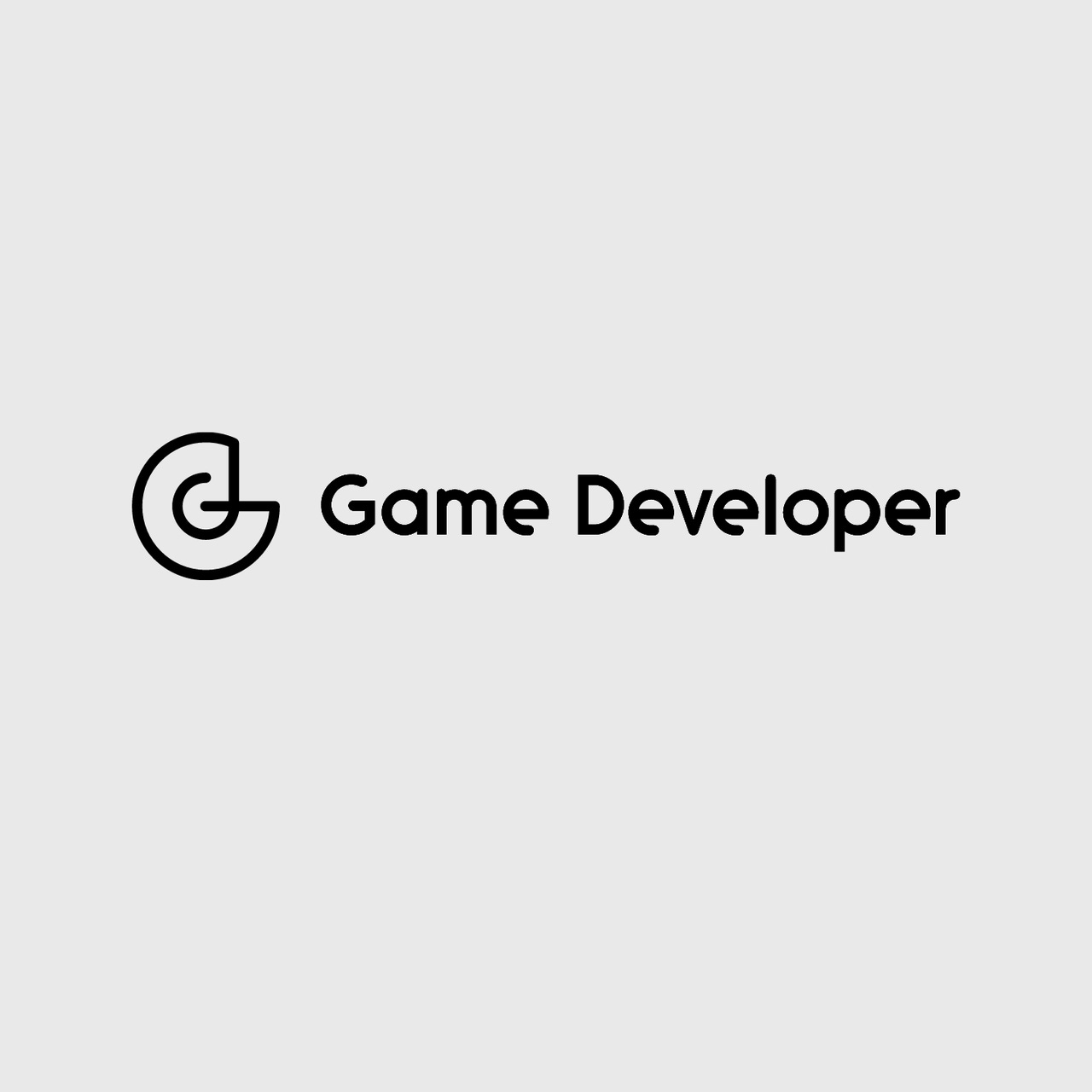
Since I had many problems finding clearly written rules about how to do stuff on Kongregate (and since I just put Stackout there), I thought I might write about that.
Connecting to Kongregate
First thing first, this might seem obvious but let me state it: you don’t need to connect to Kongregate if you don’t plan to implement its API/stats/badges/whatever.
Ok, now let’s assume you WANT to use Kongregate APIs. First of all then, you need to connect to Kongregate and tell it you’re there, so it gets ready for you. You have to connect to Kongregate as soon as you can, otherwise any API/method calls won’t work. My approach was to create a KongregateBrain MonoBehaviour, and dynamically inject it into my game at startup, in case it’s running as a webplayer. Connecting to Kongregate is as simple as firing an ExternalEval, and waiting for it to call back the result method (which is done via sendMessage). Here is the code:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25void Start()
{
// Try to connect to Kongregate.
// The gameObject.name parameter is used so SendMessage
// will look for the OnKongregateAPILoaded method
// on this same MonoBehaviour
Application.ExternalEval(
"if(typeof(kongregateUnitySupport) != 'undefined'){" +
" kongregateUnitySupport.initAPI('" + gameObject.name + "',
'OnKongregateAPILoaded');" +
"};"
);
}
void OnKongregateAPILoaded(string userInfoString)
{
// Here I set a static variable which I can
// check to know if Kongregate connection is ready
isKongregateReady = true;
// Kongregate returns a char delimited string
// composed of userId|username|gameAuthToken
// Here I just store them for easier access
string[] parms = userInfoString.Split("|"[0]);
userId = Convert.ToInt32(parms[0]); // int
username = parms[1]; // string
gameAuthToken = parms[2]; // string
}
What happens here is that, after OnKongregateAPILoaded is called, you know you can access Kongregate’s API. So be sure to check if that happens.
Knowing what’s happening behind the scenes
A good way to debug your Kongregate calls is to check what’s happening behind the curtains of your browser. To do that, just add ?debug_level=4 to the end of your game’s URL, then refresh the page: a JavaScript console (like Firebug, or the one included in Chrome Inspector) will show all the stuff that’s going on, and your stats calls.
Implementing badges
Once you’re connected to Kongregate, you can start sending badges (or achievements: they’re the same thing in case you’re wondering) when needed. Badges are considered a special type of statistic. So first of all, you’ll have to create a statistic on your game’s page, which you will then be able to access via code.
Here for example is the statistic I created to use as a “Forest World Complete” badge:
Kongregate Statistics
Now, when I want to tell Kongregate that a player has earned the “ForestWorldComplete” badge/achievement, I can simply do it like this:
Application.ExternalCall("kongregate.stats.submit", "ForestWorldComplete", 1); |
Where the second parameter is the name of the statistic I created, and the third is the value to pass – which, in case of badges, will always be 1. Notice also that I set the statistic to “Max Type”. That’s, again, due to its badge nature. No need to overwrite an achievement if it already exists, and storing it once is enough.
Where are my badges?
If you implemented badges for your game, but still don’t see an “achievements” tab appear, don’t worry. It’s how Kongregate works. I had a very hard time to find info about this, since it’s not written clearly anywhere, but achievements are not automatically added, once you implement them. You have to wait for Kongregate’s staff to, possibly, decide to implement them on their side (and they usually create the badges graphics by themselves).
So my suggestion is, if you plan to have achievements in your game, don’t rely 100% on Kongregate. While waiting for badges to be ready, add some visual feedback directly inside the game to let players know they got a badge.
Read more about:
Featured BlogsAbout the Author(s)
You May Also Like