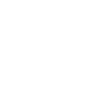
Featured Blog | This community-written post highlights the best of what the game industry has to offer. Read more like it on the Game Developer Blogs.
Line / Plane Intersection in Unity3D
How to find the intersection of a line and a plane in Unity3D without using Raycast.
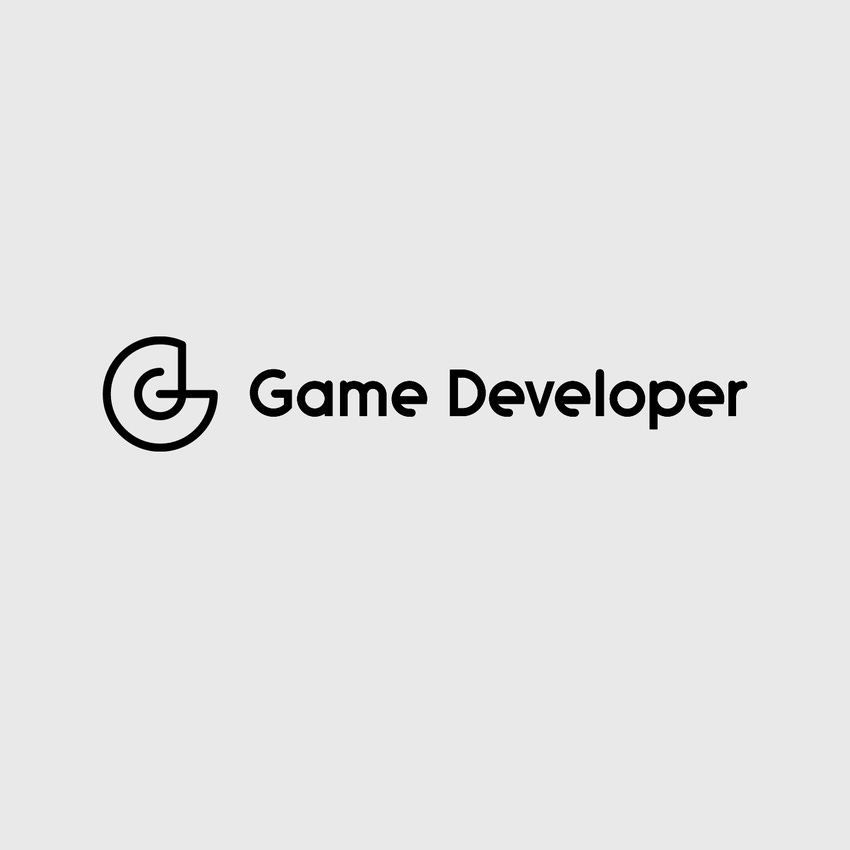
This post was first published on my personal blog.
In a recent Unity3D game prototype I needed to determine the point of intersection between a line and plane. A player would click on the screen where they wanted their spaceship to go, and I needed to work out where that actually was in the world coordinates. The line is defined by the camera point and the point on the screen clicked (as given by camera.ScreenToWorldPoint). The plane was the spaceship (assuming no up or down movement).
If the line was intersecting an object in the scene then this could be easily achieved with Unity3D's built in functions. In this case by casting a ray from the camera and finding the objects it hits (see the Raycast help page). However, my prototype is set in space, there is no object there to hit other than a small spaceship.
First, let's work out the equation of the line from the camera (point 'c') and the mouse click (point 'm'). We can get the position of the camera with var c = camera.transform.position and the position of the mouse click with:
var s = camera.ScreenToWorldPoint(Vector3(Input.mousePosition.x, Input.mousePosition.y, 100.0));
Note that in the above, the third vector parameter (the Z value) in the ScreenToWorldPoint call is the distance from the camera of the resulting point. Thus if 0 or mousePosition.z (which is also 0) is used then the resulting point is just the camera location itself. Here I have used a distance of 100 to make sure there is a good separation between the points describing the line. The general equation of a line in 3 dimensions is (for the points c & m):
[c.x + (m.x - c.x) * t, c.y + (m.y - c.y) * t, c.z + (m.z - c.z) * t]
So to find the point of intersection requires solving that simultaneously with the equation of the plane. Here is where my scenario gets easy. The plane this line intersects with in my game is the horizontal plane the spaceship is current on. That is the plane is described by:
y = spaceship.y
At some point on the line the following holds as long as the line intersects at some point:
spaceship.y = c.y + (m.y - c.y) * t
which means:
if (m.y == c.y) {
t = 0; // no intersection
} else {
t = (spaceship.y - c.y) / (m.y - c.y);
}
The case m.y == c.y denotes the case where the line does not intersect the plane. What happens here is up to you. With the value of 't' known the equation for the line can be solved to give the point of intersection. Done.
Update: Since writing, I have realised another way of solving this problem is to create an invisible plane at spaceship.y with a collider on it. Then creating a Raycast passing through the camera and mouse point will give the hit point with the plane. Although, I still use the method described above.
Contact Details:
Twitter: @JarrahTech
Facebook: JarrahTechnology
Instagram: @JarrahTech
Email: charles at jarrahtechnology dot com
Read more about:
Featured BlogsAbout the Author(s)
You May Also Like