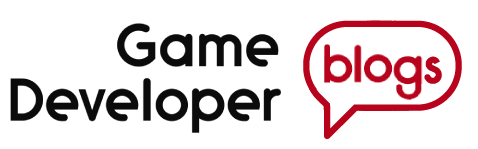
Featured Blog | This community-written post highlights the best of what the game industry has to offer. Read more like it on the Game Developer Blogs or learn how to Submit Your Own Blog Post
Finding Missing Object References in Unity
Missing references occur when a reference points to an invalid object id (may happen due to bad merge, for example). This post provides a short & simple solution for finding “missing” object references in Unity.
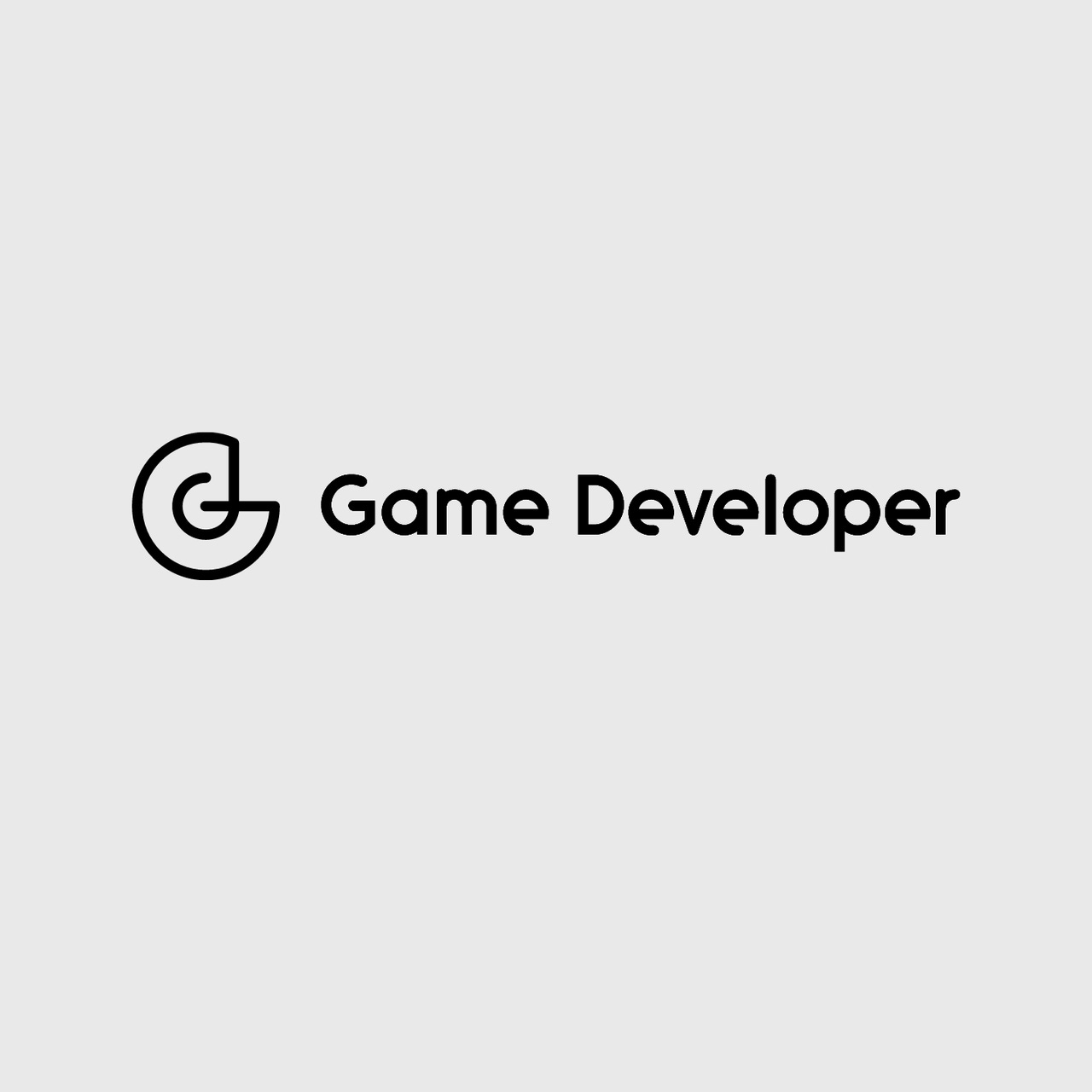
This article was originally posted on my blog at http://www.tallior.com
The post provides a short solution for finding “Missing” object references.
I plan to have more of these code posts that solve a specific problem I have faced myself; hopefully these could assist others and save them valuable time. Check out the blog for more of these articles in the future !
Missing References
A missing reference is different from no reference at all (in which case the inspector shows “None”). These are probably the result of some mixup in .meta files that results in having a link with an invalid object id. The problem is, for a large project it may be difficult to spot all these. Luckily, we can exercise some editor scripting to the rescue…
Solution
Here is the full code for finding these missing references, in case you run into this issue:
[MenuItem("Tools/Find Missing references in scene")]
public static void FindMissingReferences()
{
var objects = GameObject.FindObjectsOfType();
foreach (var go in objects)
{
var components = go.GetComponents();
foreach (var c in components)
{
SerializedObject so = new SerializedObject(c);
var sp = so.GetIterator();
while (sp.NextVisible(true))
{
if (sp.propertyType == SerializedPropertyType.ObjectReference)
{
if (sp.objectReferenceValue == null && sp.objectReferenceInstanceIDValue != 0)
{
ShowError(FullObjectPath(go), sp.name);
}
}
}
}
}
}
private static void ShowError (string objectName, string propertyName)
{
Debug.LogError("Missing reference found in: " + objectName + ", Property : " + propertyName);
}
private static string FullObjectPath(GameObject go)
{
return go.transform.parent == null ? go.name : FullObjectPath(go.transform.parent.gameObject) + "/" + go.name;
}
To use, paste this code into a new class in an editor folder, load the scene you’d like to find missing references in, and click the menu option “Tools/Find Missing references in scene”.
Any issues found will be shown in the Console (as errors).
Read more about:
Featured BlogsAbout the Author(s)
You May Also Like