Count your lines
In which I describe a fairly simple and mostly useless (but still fun) way of measuring coding progress.
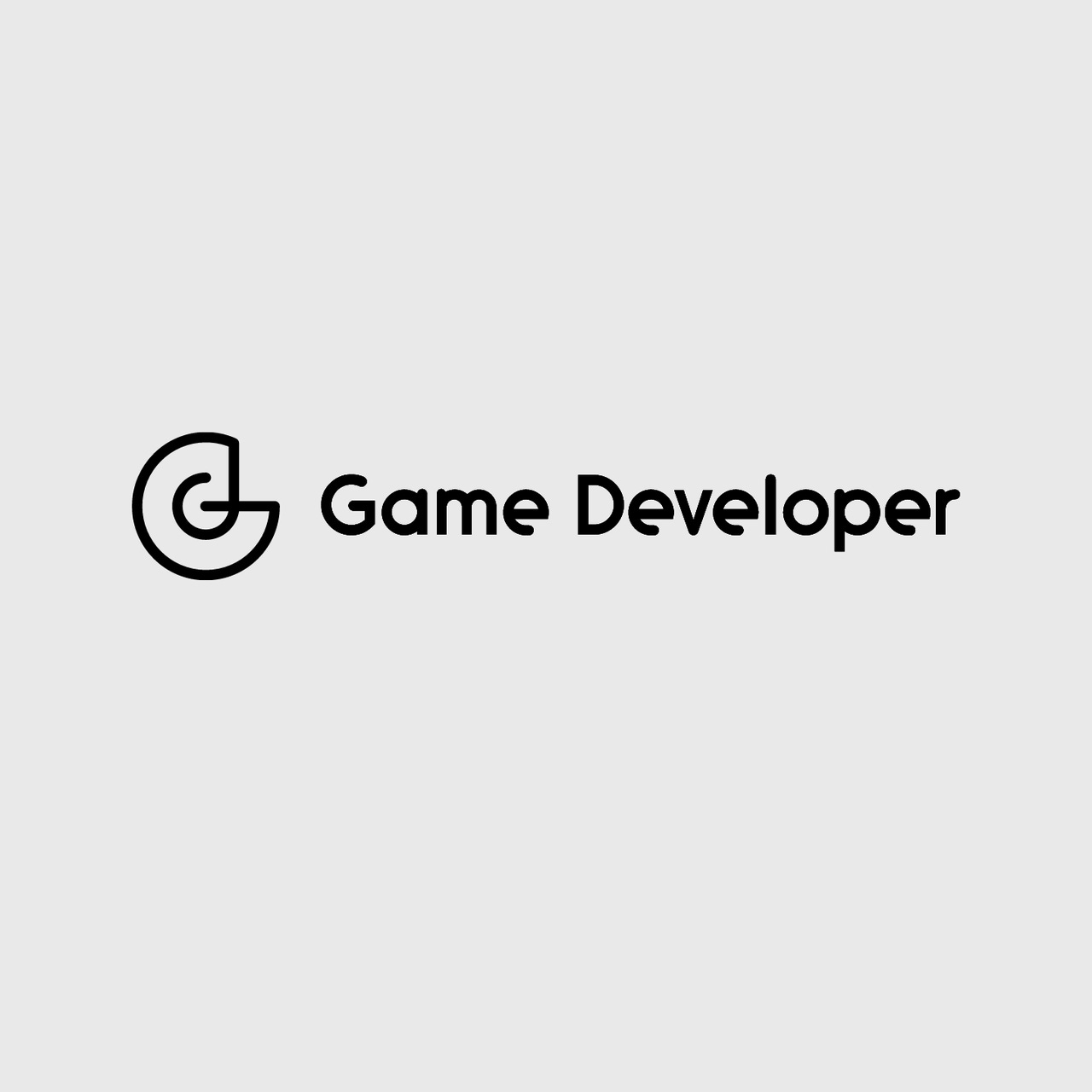
Type, type, type... Compile... Coffee... Type, type, type...
Eight hours and thirtey commits later. Blink. Stretch.
"Hmmm... How much code did I write exactly?"
It's not really a useful question to be honest. The quality of my (and your) codebase is measured and quantified by better values than the infamous "Lines of Code". Bill Gates was attributed with saying that measuring programming progress by lines of code is like measuring the progress on a Boeing by measuring its weight [not a direct quote]. And I agree. But sometimes it's still fun to weigh things.
My first thought was running the analysis tools in Visual Studio. The numbers they crunch go further than simple LOC and weren't really that interesting. My second thought was google. But alas, too many results and not enough time to go through them. It was then that I realized. Hey, let's reinvent the wheel!
With that in mind I started a new console project (this is going to be written in C#) and decided to being with the simplest thing possible. Checking if I can read all files under my current directory and output how many code files there are.
public static class Program
{
public static void Main(string[] args)
{
var root = Directory.GetCurrentDirectory();
var paths = Directory.GetFiles(root, "*.cs", SearchOption.AllDirectories);
Console.WriteLine("Found {0} code files", paths.Length);
}
}
That works. Note in particular that we're searching just for ".cs" files. And under all directories.
Next we need to actually count all lines in the files.
var line_count = 0;
Console.WriteLine(":: Counting Lines of Code...");
foreach (var path in paths)
{
using (var reader = new StreamReader(path))
{
while (!reader.EndOfStream)
line_count += 1;
}
}
Console.WriteLine("Found {0} lines of code in {1} files", line_count, paths.Length);
And that should be it, except it isn't. Our little program will now count all lines in all files. ALL lines. I don't want all lines. I want to count code lines. But wait, what do I mean by code? For our purpose with this tool we'll take it to mean any line that is neither empty nor a comment. To do this we'll introduce a new method to query whether a given line is considered for counting.
public static bool ValidateLineIsCode(string line)
{
if (string.IsNullOrEmpty(line))
return false;
var unspaced_line = line.Replace(" ", "");
if (string.IsNullOrEmpty(unspaced_line))
return false;
if (unspaced_line.Length == 1)
return false;
var ch = unspaced_line[0];
if (ch == '/')
return false;
return true;
}
And yeah, it's not perfect but for my coding standards it's pretty good and gives me a decent metric (albeit a useless one) for LOC.
It was always summer and the future called; We were ready for adventures and we wanted them all
About the Author(s)
You May Also Like