Porting game to the Unreal Engine 4
In this article I'll be talking about my experience of game porting from Marmalade SDK to the Unreal Engine 4.
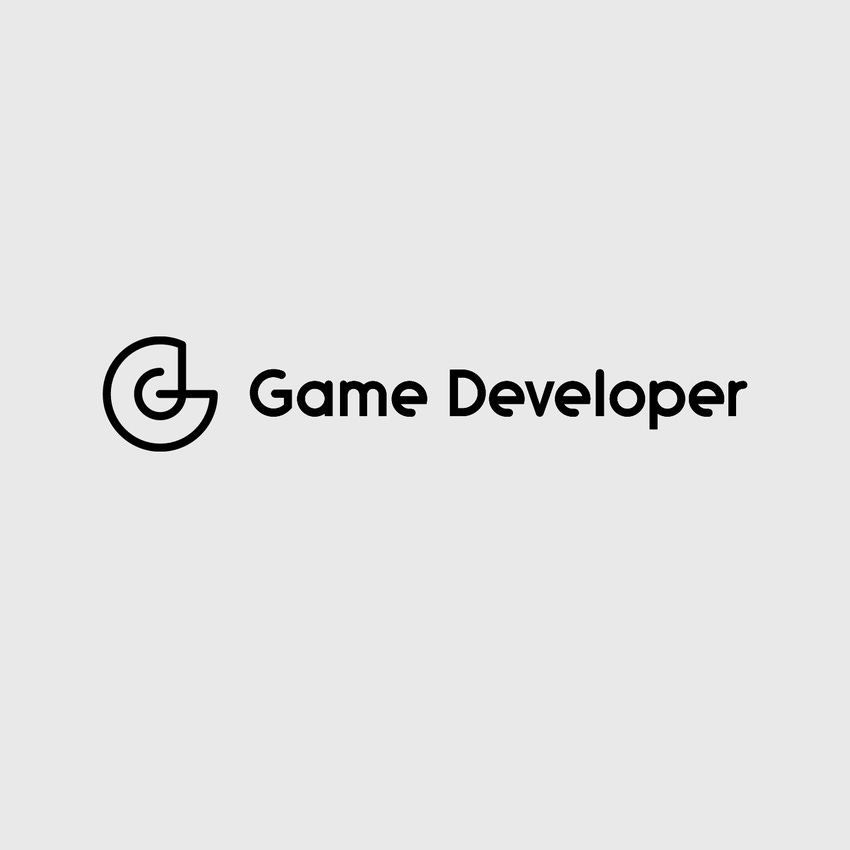
Hi everyone!
My name is Andrew - I’m an independent game developer.
Currently, I've been making a UE4 assets and developing my own games on Unreal Engine 4 tech.
About Bring Them Home
This is Stylized low poly puzzle game in Sci-Fi setting.
Game has 50 levels. In general - combinatorial puzzles.
Player is control the Cosmic drone, which interacts with astronauts.
The main goal of each level – evacuate all astronauts from space platform (the level).
All cosmonauts have different abilities: some you can pick up and drag, others can push the neighboring astronaut. Also there is a fragile astronaut who cannot be dropped from a height. It's a deep remake inspired of one cool puzzle game that i've played in early 2000.
Game has contain about one hour of special made soundtracks in space ambient style specially composed for the game.
What i have at start
Initially – game was used the Marmalade SDK tech for iOS and Android platforms.
Marmalade SDK – is not a game engine, it is rather cross-platform API. There aren't specific entities like Actor, Camera, level, etc
Marmalade SDK has been supported two programming languages – C++ and Lua and next platforms: iOS, Android, PC and Tizen.
There were three factors that triggered our rejection of mobile versions:
Shortly after the game's release, Marmalade was closed and my license expired.
Project has weak monetization. Although the reviews of the project were positive and the score above 4.5 points, the return was not encouraging at all, and we had no bags of money for traffic.
We tried different methods to improve of touch control, but have not reached desired responsiveness of control on the touchpad.
As a result, we removed all mobile versions and stopped supporting them.
But I didn’t want to leave project: most of the reviews were positive.
Therefore, I decided to port a game to Unreal Engine 4!
What I have:
C++ code, written under Marmalade SDK specification
Touch screen Control
Game assets in sources
Levels in XML format
A self-written level editor that output an XML file describes the level structure with all the necessary data
GLSL Shaders
4 languages of localization
Inability to compile a working build
Let’s start job!
Things that had to be done from scratch:
Save/Load system, controls
Pawns, Camera
Particle system
Assets upload
Materials
Level Converter from XML to UE4
Reimport all 3d models and assets to UE4, etc...
Significantly refactored
I had to cast source C++ code to UE4 Types (FVector, FTransform, UOBJECT, etc...)
It also required the addition of a BlueprintImplemantableEvent so that C ++ code can send a message to Blueprint child class. For example, when all astronauts are evacuated from level and C ++ call the LevelComplete event
Adding BlueprintCallable so that Blueprint can call and use C++ code. For example, to calculate a player’s rating. The calculation method itself is written in C++
Creating a level structure as sublevels and completely new camera logic.
Minimal edits
The least changes were in 3d models – just some fixes on the little things
After doing preliminary work with the code and assets, I started transferring levels from the the Marmalade SDK version.
Each level was presented by two XML files. The first described the game structure of level and objects like transform, properties, type, etc.
The second XML file is describes decorative elements – the location of flashing lamps, fans, antennas. Decorative elements don’t affect the gameplay, but give visual improvements of the level:
A basic actor BP_AstroBase contains the necessary data structure from the Marmalade version. All of 4 astronaut’s classes and other dynamic objects, buttons, conveyors, teleportators, elevators, were inherited from it.
I didn’t need to come up with the data structure again. Unless I removed all the non-essential, mostly cosmetic variables and data that were necessary for the Marmalade SDK to work.
Then I’ve created an actor for decoration. It contains all types of decor at the levels, draws the model necessary at the moment and carries out actions with it.
The converter read two XML files, parsed them and spawned the required actors in UE4. Final step - it’s just add StaticMesh with level geometry.
After finalizing the converter, transferring all 50 levels took 1.5-2 hours.
The Game Architecture
The game has 3 types of Pawns:
Main Menu Pawn. Used in the main menu to control click on the buttons: Start Game, Options, and Help etc. Entirely on Blueprint
LevelsPawn. Handles scrolling between planets and level selection. Entirely on Blueprint
PlayerPawn. The base class is written on C++ and contains all the gameplay logic of the game, transferred from Marmalade SDK version.
GameInstance
Contains the entire system functionality of the game: loading/unloading levels, saving and loading the game state, rating calculation, game variables that need to be transferred between levels, for example, common game timer.
It is written in C++ and has a Blueprint child.
The levels are made as sub levels approach. i.e. this is levels streaming. This approach has two significant advantages:
No need to make a separate thread for continuous music playback
Saving build size. All common elements used in most levels are persistent and don’t require duplication to each level. These elements include: MusicPlayer, Background texture, spaceships that fly in the background, PostProcess Volume and flying stars FX.
The game Logic cycle of the UE4 we can described:
Waiting for events from the player in Pawn(level selection, calling options etc)
Unloading the current level(any level is always loaded)
Saving current data(level number, timer, etc)
Loading the new level
Transfer of control to Pawn
Actor’s Tick() Sync
In the Marmalade SDK version, all logic calculations, update, render, etc. executed inside of MainLoop with a strong specified sequence.
In UE4, each actor executes Tick () asynchronously. The main logic is in PlayerPawn, due to which there was a situation where some of the actors could execute their Tick before it was executed by PlayerPawn, and the other part after.
To solve this small problem, it is convenient to use the AddTickPrerequisiteActor() method. So we directly indicate the sequence of ticks in different actors.
Having set all actors such a condition (except PlayerPawn), we put PlayerPawn at the top of the list and it will always tick first.
I tested with AddTickPrerequisiteActor and without it I didn't notice a significant difference. Therefore, in the final version of the game this solution is removed.
Teleport FX
The game has teleporters and a teleporting effect created especially for them. In the version on the Marmalade SDK, it was implemented using a self-written particle system and consisted of particles flying from start to target along a path close to a parabola.
As many teleports as you like can be performed at the same time.
In UE4, the fastest solution was to use InstancedStaticMeshComponent.
Firstly, I already had the logic of calculating the trajectory.
Secondly, ISMC is drawn in 2-4 (depending on the material) draw calls.
All that was needed was to adapt the logic for updating the ISMC transforms. In UE4, there is also a HierarchicalInstancedStaticMeshesComponent that supports LODs, but in my case, lods support was not required.
PlayStation 4 improvements
For PlayStation 4, I wanted to release a better quality version of the game:
Adding skeletal astronaut’s animation
Two new soundtracks
Dynamic Ambient Occlusion
The VFX of the evacuation stage
Additional 8 languages of localization
Additional VFX
Deferred UE4 renderer
The timing
It took me exactly 30 days for 12-14 hours.
Frankly, after such a crunch, I slept for a couple of days and did not respond to any external signals.
There were no difficulties and significant problems during the transfer process, and I cannot highlight anything special. Perhaps, only the variety and mass of small tasks that needed to be done and not forgotten.
Trailer
I decided to add a couple of words about creating a story trailer to this article because it was created for the UE4 version. The version on the Marmalade SDK had only a gameplay trailer.
I believe that it positively affects the player’s perception of information about the game at the initial stage. The trailer tells a short story and smoothly leads to actions in the game and helps empathize with the fate of little heroes.
The trailer was created by another person, for which he is very grateful.
Despite the fact that he didn't have too much experience in UE4, he was able to quickly figure out all the necessary tools and assemble the trailer in Unreal Engine 4 using sequencer and other tools.
Initially, there was only a storyboard, drawn by me by hand - in fact, the key frames of the trailer:
Next, we discussed the scenario of what needed to be obtained at the exit and how long.
In a couple of weeks, a draft cinematic was ready without effects, textures, sound, light and other beautiful things. It contained the final timings, foreshortening and animation.
After a couple of minor edits, he went to the composer, who wrote the soundtrack for the video. At the same time, the cinematic was transferred to UE4 and the work on finalization began - light, effects, materials, etc.
After another 7 days, I received the final project in UE4.
I had to correct the gamma, because it was too warm and, in my opinion, was weakly associated with space, and also fixed Camera Depth of Field bugs.
A few days later soundtrack from composer arrived and I put together the final version of the trailer.
All the work from creating a storyboard to the final trailer took 23 days.
After release
More than 3 months have passed since the release on the PlayStation 4, and I can look at the game with a somewhat abstract look and draw certain conclusions:
Shorten trailer duration - Even by changing the storyboard. About 15-20% of it is cut without loss of idea and motion design.
An old school tutorials showcase - Training levels are the first 10 levels. They do not contain puzzles, only demonstrate gaming capabilities. This is a very very outdated approach. Ideally, it must be replaced to the tips for each level.
Astronauts haven’t eyes - Despite the fact that the astronauts are animated and are no longer perceived by mummies in space, it was necessary to add eyes to convey emotions - for greater association with living heroes.
UX - After completing the level, Level Complete appears. Unfortunately, this happens in a trivial way - just an inscription appears and that's it. The player doesn’t have a sense of achievement. It was necessary to add animation and major sound effect to emphasize the importance of achievement.
Sequence of levels - The first levels are extremely simple and the play testers said that playing is boring. It becomes interesting to play only after about 22-23 levels. It was possible to rearrange the levels in places, giving a challenge already at level 13 or 14 in the form of a rather complicated puzzle. Unfortunately, this would require a lot of extra work.
Conclusion
It was an interesting experience and I would like to thank all the people who somehow participated in the creation of the game!
Special thanks to Sony Interactive Entertainment for the opportunity to release the game on the PlayStation 4, giving all puzzle lovers to play it.
Big thanks to Epics games for the superb Unreal Engine 4 tech!
Thank you for your time, good games to everyone!
Read more about:
BlogsAbout the Author(s)
You May Also Like