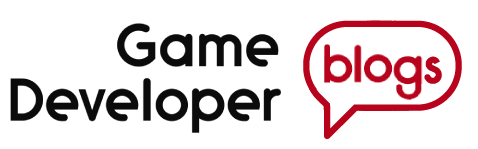
Featured Blog | This community-written post highlights the best of what the game industry has to offer. Read more like it on the Game Developer Blogs or learn how to Submit Your Own Blog Post
Secrets of the Unity Animation View
The Unity Animation Window is one of the most powerful, but also most overlooked features of Unity. Learn how to leverage the full power of the Animation View, for programmers and non-programmers alike in this post by components developer Will Hendrickson
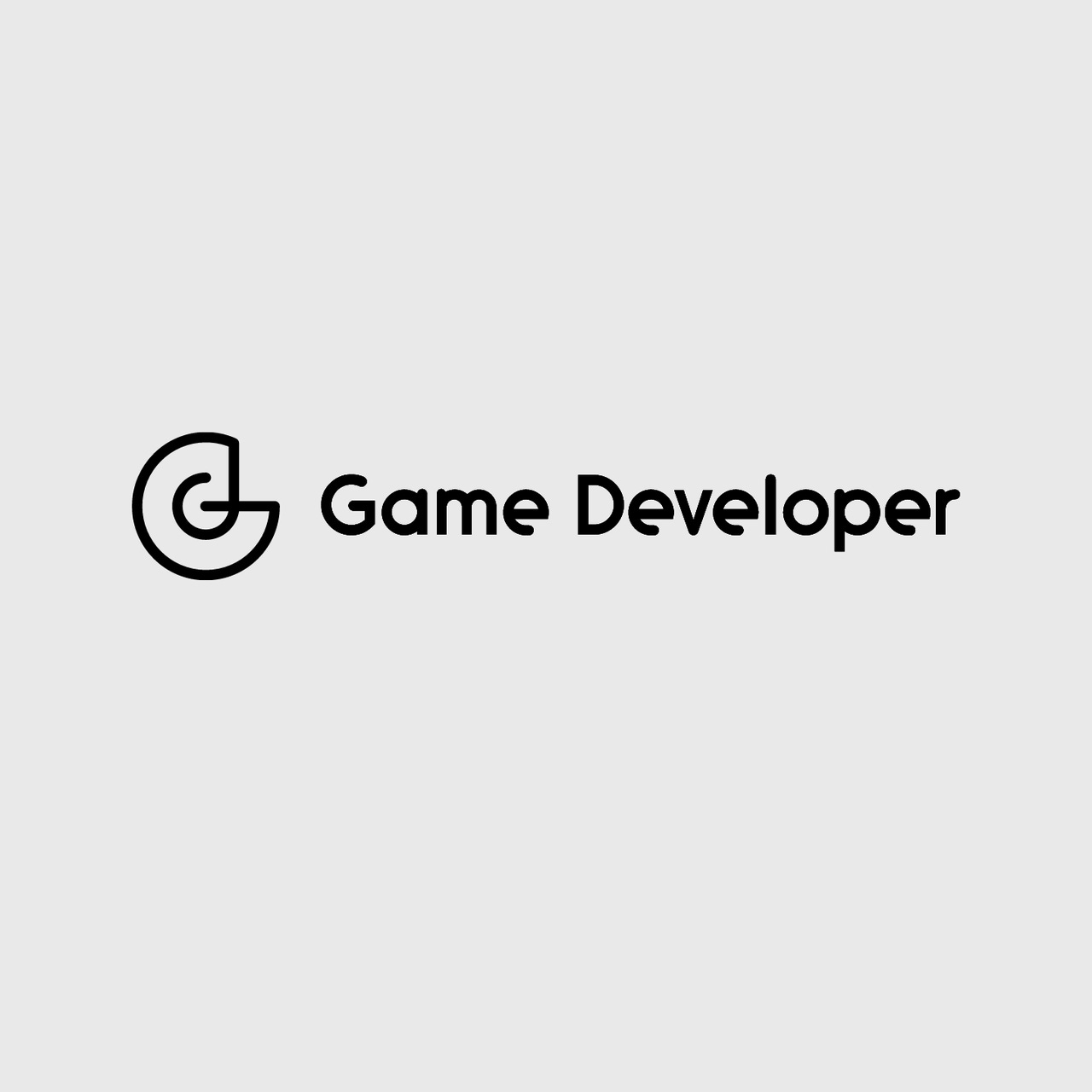
While developing games with Unity, I was always looking for ways to build them faster, smarter, and with less repetitive effort. I found that, but only later after trying a number of things that didn't work, and I'd like to share some of my experience with you.
My first instinct was to use a visual scripting system to simplify the task of developing gameplay. This worked, in the beginning, but as the complexity of the game or even a single feature increased, the visual scripting systems became less and less adequate, until eventually they simply couldn't handle the complexity of the overall game. The bottom line is, these are great for simple things like infinite runners or basic physics games. But, if you want something more complex, you need something more powerful. There is scripting, of course, and complex scripts can take care of everything. But, what if you only want to have to create some basic script functionality?
What I found was that I often wanted a series of events to occur in a particular order. Play a sound, then spawn the bad guys, and finally create a boss. Play an animation, a sound, and a bit of code to change the scene. Move the platform into position, play some particles and light, and wait for the player. Etc...
I remembered, from reading the Unity doc, that script functionality could be called from the Animation view. And, I began finding scripts that had useful functions that I could call and send info to, and stringing them together into a Logic Sequence.
It all depends on the way you set up your prefabs. For example, did you know that you can create advanced things like security cameras and area spawners and even shadows on the indie version of Unity without scripting or using visual logic editors like Play Maker? You can create entire games without programming already by getting Asset Store packages, but most people don't realize you can customize the flow of the game using the Animation view.
Many games through gaming history and even today use timeline-based sequences to control the flow of the game. Unfortunately, many developers are unaware of the power of the Animation window and some even spend a large amount of time and money developing custom editor tools!
Unity comes with a large number of built-in features that can be developed without scripting to create interesting simulations. By using the Standard Assets package and the Animation window, you can create basic exploration and puzzle games without scripting. With some practice, these can become quite fun especially when using cool Unity features like Interactive Cloth-based soft body physics or springs and hinges.
Ok, enough about the virtues of the feature, let's learn how to do it!
Example, Day/night cycle:
1. Open the latest version of Unity. As of this writing, 4.3.
2. Click Window -> Animation
3. Dock it to the bottom of the screen.
4. Create a directional light, name it something appropriate
5. Click on the small gear next to the blue book in the top right of the Transform component, and click Reset
6. With the directional light selected, move your mouse over the Scene view and press F
7. Then press E
8. Then, while holding [ctrl] on Windows/Linux or [CMD] on a Mac, grab the green ring and rotate it until the y = -90
9. Click Assets -> Import Package -> Lens Flares
10. In the inspector, Click Add Component -> Effects -> Lens Flare
11. Assign a Flare to the Lens Flare component by clicking the dot to the right of the value. This will represent the sun.
12. Make sure "Directional" is checked
13. Set up the layers the flare should ignore. These should not be the colliders that you want to block the sun such as terrain, buildings etc
14. You may also tweak the starting color and brightness of the flare, as well as the light itself. This should be set up nicely until it looks like a sun on the horizon. No SkyBox. We will animate the camera's "Clear Color" property instead, to represent sky color and add some particle clouds if we want. This will create a nice procedural and semi-volumetric sky effect that is good even for flying games.
15. In the top left corner of the Animation Window, click the small red recording button, and save the new Animation clip in an appropriate folder in your project
16. Click the "Curves" button, and add the following:
A. Transform -> Rotation
B. Lens Flare -> Brightness
C. Lens Flare -> Color
C. Directional Light -> Color
17. Select "Rotation"
18. The "Scrub Bar" is the time line at the top of the Animation window, clicking in it sets the Current Frame, indicated by a red line. By holding [CTRL] or [CMD] for mac, you can zoom out by time. By holding [SHift] you can zoom by value.
19. Zoom out by time and set the scrub bar to 30 seconds
20. Change the rotation to 90, -90, 0. This creates a new keyframe at 30 seconds and records the new values automatically.
21. Set the scrub time to 60 seconds
22. Change the rotation to 180, -90, 0
23. In similar fashion, animate the remaining curves.
24. In the Animation window, press the small play button. This will not play the game but will instead allow you to preview the sequence.
25. Change until satisfied. You can make a night time cycle by adding more keyframes and setting dark blue light and sky color, and flare brightness to 0. If a property gets animated and you don't want it, right click it in the Animation window and click "Remove Properties"
26. Unity will automatically add properties and keyframes while the red record button is active! Toggle it if you want to make changes to a default or non-animated property!
27. When you're done, repeat the process on the camera to animate the clear color, and set the camera to clear to solid color. This represents your sky color, so it should be brightly colored for dawn/dusk, sky blue for the day, and dark navy blue during the night if you want to have a lush or earth-like atmosphere.
You can slow the speed of the animation by spreading out the keyframes, creating a longer cycle. Zoom out farther using [CTRL] or [Shift] if you need more room.
Well, great, now we have a day/night cycle but it doesn't really do anything with gameplay, it just makes the scene look pretty. Next, we'll go over how to use this with scripts. You can use your own, or download them from the internet/Asset Store. In this tutorial, I'll go over some very basic C# that will help you plug in gameplay with the Animation window, allowing you to literally animate or timeline anything. This is great for things like spawn waves, puzzles, or anything at all that moves or works based on a function of time.
Example, Night zombies:
1. Create some zombie enemies and assign them to a prefab. This is the subject of a different tutorial! Again, you can just get some AI and zombie models from the Asset Store and online.
2. Create a new script, call it something clever like ZombieSpawn
3. Fill the script as such:
using UnityEngine;
using System.Collections;
public class ZombieSpawnExampleScript : MonoBehaviour {
public GameObject zombiePrefab;
public GameObject[] spawnPoints;
public void SpawnZombies () {
foreach( GameObject spawn in spawnPoints) {
Instantiate(zombiePrefab, spawn.transform.position, spawn.transform.rotation);
}
}
}
Now, assign this to an object, assign your zombie to the Zombie Prefab property in the inspector, and add a new Animation to it like we did with the sun and camera. Optionally add zome spawn points, otherwise they will spawn at the position of this object. Finally, set the scrub bar to the desired time of the first Zombie spawn, and notice the small, lighter gray bar just underneath the scrub time. Right click in this area to add a new event, and add SpawnZombies as the function. For more advanced users, you may pass a single parameter, for example allowing you to spawn any enemy and not just a single zombie prefab.
The Unity Animation view (not 'Animator' which serves a different purpose) is the single most powerful time-lining tool you have when developing games with Unity. The key is in prefab setup, and understanding the capabilities of the components that come with Unity. For example, did you know that the Standard Assets -> Scripts package comes with an excellent trigger activation functionality? This, combined with animations and physics components such as constant force and constrained rigidbodies or spring/hinge joints you can create a wide variety of gameplay and puzzles with very little or no scripting. It all comes down to the setup of your components, and using them on different prefabs wisely. And remember, Animation is for designers too!
Read more about:
Featured BlogsAbout the Author(s)
You May Also Like