Shoot SFX - Procedural Audio
In this tutorial I explain how to make SFX of shoot without sound file.
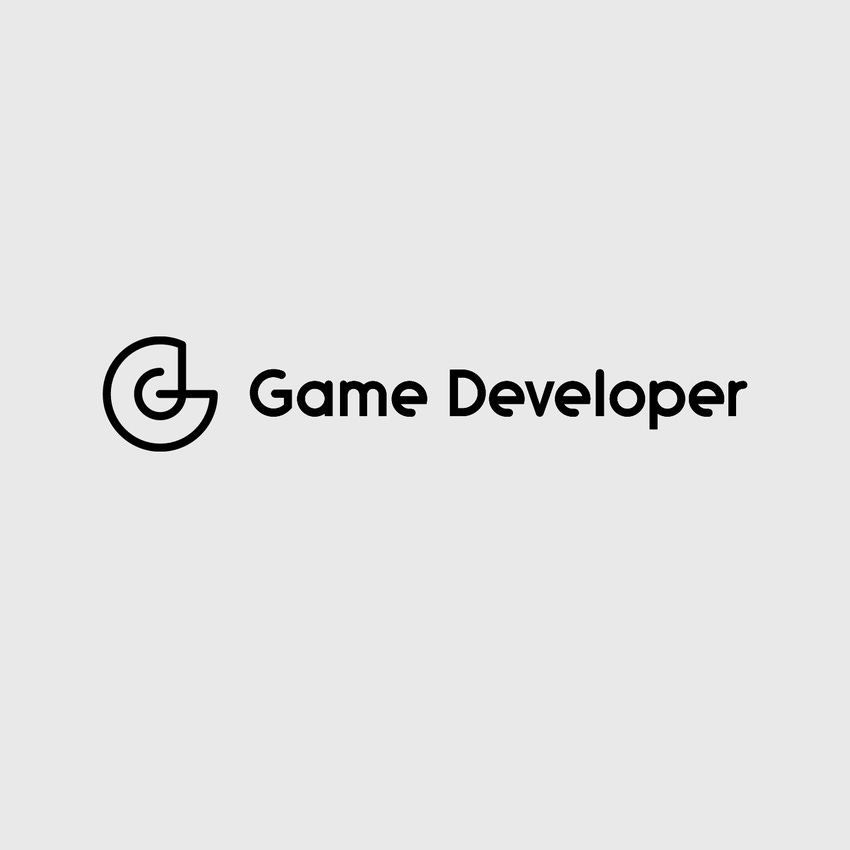
In this tutorial I explain how to make SFX of shoot without sound file.
Prerequisites:
1. Basic of C#
2. Basic of Pure Data
1. Create pd patch
Main point of our patch is sound generator. There are 3 generators. First is [noise~], which is white noise generator. We have to cut bands from noise and control volume.
I prepare to compile in heavy compiler, objects like [r volMaster @hv_param 0 2 1] are necessary (see https://github.com/enzienaudio/hvcc/blob/master/docs/02.getting_started.md. Let’s look inside [pd band]:
[pd high] and [pd low] are similar. High has high pass filter and low has low pass filter.
ADSR is envelope control of filtered noise signal.
For now we made gun shoot sound. What about sci-fi guns? Sci-fi weapon sound can generated from sine wave or sawtooth. You have to set high frequency and go to lower.
Whole patch should like this:
This patch compile using heavy compiler https://github.com/enzienaudio/hvcc.
2. Unity
Create GameObject and add heavy script. We need control script (OnScreen.cs).
using System.Collections; using System.Collections.Generic; using UnityEngine; public class OnScreen : MonoBehaviour { //private Hv_UnityGuns_AudioLib audioLib; // Control gun shoot public void GunShoot1(){ Hv_UnityGuns_AudioLib script = GetComponent<Hv_UnityGuns_AudioLib>(); // Change parameters and listen difference. Sin and saw tooth generators are muted. script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volosc, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volphasor, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volband, 1); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Band, 500); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Randomband, 130); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Vollow, 1); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Lop, 300); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Randomlow, 40); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volhigh, 0.01f); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Release, 120); script.SendEvent(Hv_UnityGuns_AudioLib.Event.Play); } // Control second gun shoot like above public void GunShoot2(){ Hv_UnityGuns_AudioLib script = GetComponent<Hv_UnityGuns_AudioLib>(); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volosc, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volphasor, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volband, 1); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Band, 600); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Randomband, 250); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Vollow, 1); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Lop, 200); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Randomlow, 60); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volhigh, 0.1f); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Release, 90); script.SendEvent(Hv_UnityGuns_AudioLib.Event.Play); } // Control Sine wav shoot. Noise and saw generators are muted. public void OscShoot() { Hv_UnityGuns_AudioLib script = GetComponent<Hv_UnityGuns_AudioLib> (); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volosc, 0.6f); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volband, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volhigh, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Vollow, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volphasor, 0); script.SendEvent (Hv_UnityGuns_AudioLib.Event.Play); } // Control Saw tooth generator. Like above, noise and sine wav generators are muted. public void SawShoot() { Hv_UnityGuns_AudioLib script = GetComponent<Hv_UnityGuns_AudioLib> (); script.SetFloatParameter(Hv_UnityGuns_AudioLib.Parameter.Volphasor, 0.6f); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volband, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volhigh, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Vollow, 0); script.SetFloatParameter (Hv_UnityGuns_AudioLib.Parameter.Volosc, 0); script.SendEvent (Hv_UnityGuns_AudioLib.Event.Play); } // Use this for initialization void Start () { } // Update is called once per frame void Update () { } }
To play shoot sound you call function GunShoot1(), GunShoot2(), OscShoot() or SawShoot(). I made GUI to play that.
3. End
Project with pd file you can download from my github: https://github.com/kretopi/UnityGuns.
Read more about:
BlogsAbout the Author(s)
You May Also Like