LibGDX in Depth series - Entry 01
This blog series is just me talking to myself about how libgdx works. This will not be about how to write a game but about how LibGDX works on the inside, which will help me and you ofcourse to expand this awesome engine. disclaimer : I'm a noob
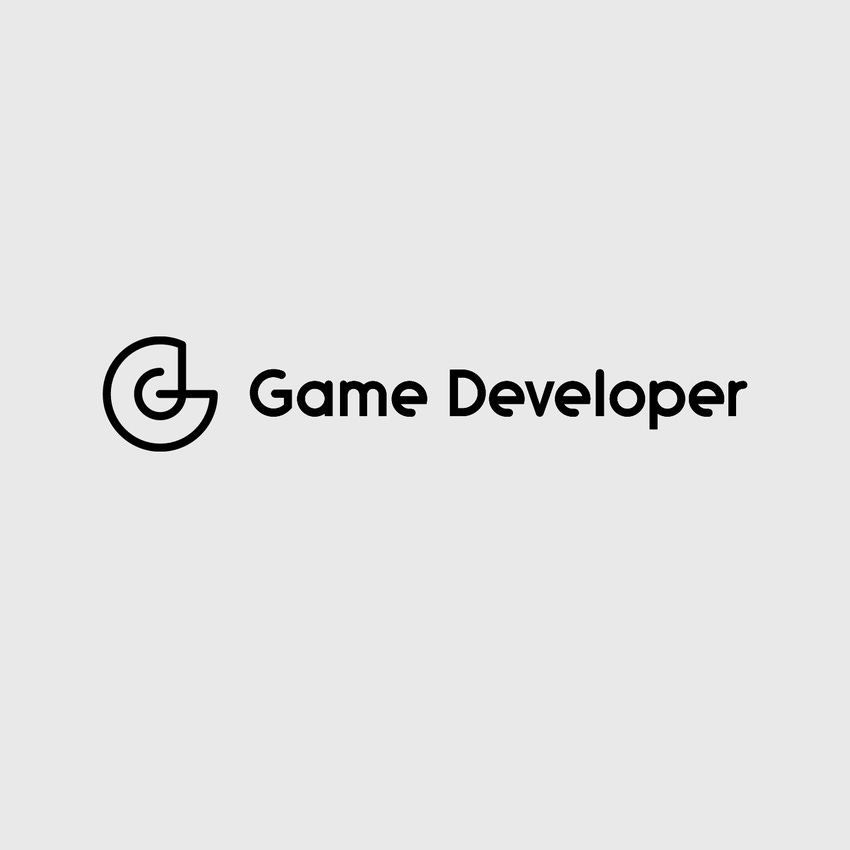
Blog orginally from jaykalabs.com
This is the first entry towards my exploration into the world of Computer Graphics and OpenGL through LibGDX. I will record my musings and ideas and what i understood about LibGDX in this series, which will serve as a reminder to me and a tutorial to you. Please note that this series will be slightly difficult for total beginners to OpenGL.
A New Beginning
I'm not going to discuss in detail about how to setup your first LibGDX project. You can find information regarding that here . Desktop and android project has to created as a minimal requirement. I have named my project "LibgdxTut01" and am using LibGDX 0.9.9.
Into the Depths
Our objective now is to get the below image as output. I'll be using the desktop project for that.
We'll not need any asset now, so delete the 'assets/data' folder inside android project. We'll be using OpenGLES 2.0. Hence we have to set the LibGDX configuration to use OpenGlES 2.0. Below is the snippet from my Main.java (desktop project).
LwjglApplicationConfiguration cfg = new LwjglApplicationConfiguration(); cfg.title = "LibgdxTut01"; cfg.useGL20 = true; cfg.width = 480; cfg.height = 320; new LwjglApplication(new LibgdxTut01(), cfg);
In OpenGL everything we render is a series of triangles. Even the most complex scene from crysis is just a series of triangles with textures & etc. A group of these triangles is called mesh. The above triangle is a mesh with three vertices. Each of these vertices can have many attributes like color, texture coordinate , normal , tangent. For now i'll use only color. You can see that i have give pink,red and white color for the vertices. See how it has smoothly blended. We have to sent the position of the vertices and color of the vertices to graphics card, so that it can draw it. LibGDX provides a convenient class aptly named Mesh. Creating a new mesh now I add the below code inside Create function of libgdxtut01 class.
mesh = new Mesh(false, 3, 3, VertexAttribute.Position(), VertexAttribute.ColorUnpacked());
We are telling LibGDX that we need 3 vertices and we have 3 indices (number of triangle coords). You'll understand indices as we move on. For now I'll just tell that triangle has 3 vertices,3 indices. Square has 4 vertices, 6 indices(2 triangles). Pentagon has 5 vertices 9 indices (3 triangles).
Next in the constructor is VertexAttributes. position attribute requires 3 floats(XYZ) and color unpacked required 4 floats (RGBA). So in the constructor we are telling LibGDX that first 3 floats are position and next 4 are color of a vertex. so in the below code. (-0.5f, -0.5f, 0) are position of '0'th (zeroth) vertex and (1, 0.4f, 1, 1) is the color. The next (0.5f, -0.5f, 0) and (1, 0.2f, 0f, 1) corresponds to next vertex.
mesh.setVertices(new float[] {-0.5f, -0.5f, 0, 1, 0.4f, 1, 1, 0.5f, -0.5f, 0, 1, 0.2f, 0f, 1, 0.5f, 0.5f, 0, 1, 1, 1, 1}); mesh.setIndices(new short[] {0, 1, 2}); Next comes the shaders. OpenGLES 2.0 uses what is called as shaders for shading the meshes. There are many tutorials about what shaders are. You can refer here also. I've written my vertex and fragment shaders in files inside "assets/shaders" folder so that it need not be edited easily.
shader=new ShaderProgram(Gdx.files.internal("shaders/vertex.vert"),Gdx.files.internal("shaders/fragment.frag"));
The ShaderProgram class will automatically compile,attach and link the shaders. Check shader.isCompiled() to check for errors. ShaderProgram class makes writing shaders and passing values very easy. Now to render the mesh add the following code to render loop.
Gdx.gl20.glClearColor(0.2f, 0.2f, 0.2f, 1); Gdx.gl20.glClear(GL20.GL_COLOR_BUFFER_BIT); shader.begin(); mesh.render(shader, GL20.GL_TRIANGLES); shader.end();
We clear the framebuffer with gray color. shader.begin informs OpenGL to use the shader program for rendering all meshes upto shader.end. mesh.render creates the draw call for rendering. Run the desktop java project and you should see the above output. You can find the source code for this entry in github. If you have any doubts feel free to comment.
We'll look in detail into how Mesh class and Shader Class are implemented and how it interacts with GL context in next few entries.
Read more about:
BlogsAbout the Author(s)
You May Also Like