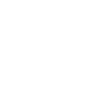
Featured Blog | This community-written post highlights the best of what the game industry has to offer. Read more like it on the Game Developer Blogs.
iOS: Thoughts on Objective-C For Games
iOS:Objective-C has opened new markets for devs, and is increasingly expanding as development restrictions get lifted or lightened. A visual artist cum hobbyist programmer gives his insights.
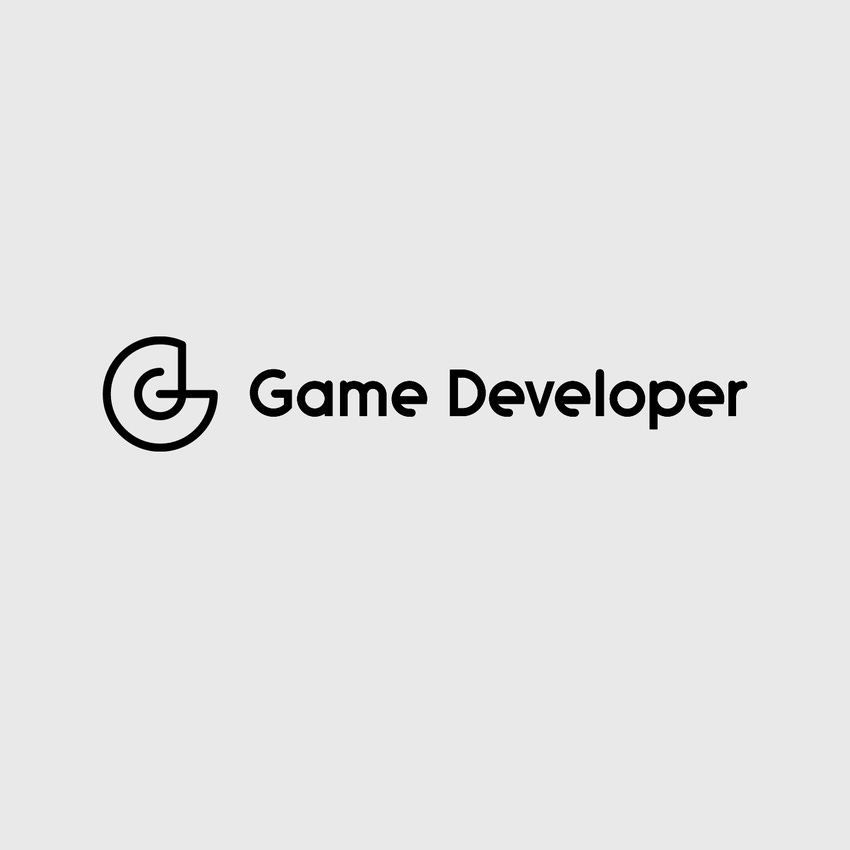
The iPhone and more recently iPad have opened up entirely new markets for name studios and indie developers to share their creative visions and products and reducing the concerns of overhead, distribution, and physical space considerations that came with, up until recently, almost all gaming products. The App Store has likewise provided a central clearing house for devs to distribute their product, for a 30% cut of all revenue. This has been done before with systems like Steam, but Apple’s App Store became focused entirely on their mobile iOS platforms. Things such as the Android Market and other stores have popped up; however, Apple was one of the first players on the scene and have secured themselves a sizeable market share of digital downloads for mobile devices.
This is all well and good, but there are things, I, as a novice Objective-C (henceforth in this article as Obj-C) programmer, found to be challenging with Objective-C versus other programming languages (Python, C/C++/C#, V.Basic) that I have taken the time to sit down, learn, and take classes on. On the surface, Obj-C is a superset of the original C language integrated with a programming language built by Steve Jobs’ company in the interim years when he was ousted from Apple, NextStep, which have added a wrinkle to the cloth of latter day C-programming.
By the same token, to create fully featured games, this has necessitated the development of engines and wrappers for developers who want to create serious games beyond simple word scrambles, hangmen, and pong types, simply in the interest of saving time and resources because of the challenging wilderness of Obj-C. When starting out early on, one will learn that following Apple’s Model-View-Controller (MVC) structure is often the best way to go and will save a lot of heartache. Unfortunately, I like many others, tried to resist and got placed well into the labyrinthine world of debug hell numerous times. View controllers (which I will discuss later on ) will easily become your best friend.
Obj-C also has the benefit and curse of being paradoxically fairly loose, being that there are multiple ways to do things, and bears extremely rigid syntax (using @ signs for strings will definitely throw you the first few hundred times), programming standards (look up Touch Classes to get my drift… if you use conductive touch class methods and forget even one, you will likely find your program rejected) and some internal compatibility inconsistencies as the language evolves and older, less efficient features get culled. Another issue, but thing I later came to like, was the requirement to bracket methods. Not the classic curly braces, but methods in Obj-C frequently have their calls, and various modifiers and internalized variables that come at varied levels of [] bracketing. Whereas in C/C++/C#, one might simple call the class or method after defining it earlier either in a .h file or earlier in the file in question and simply invoke it, as such from a purely programmatic perspective – IDEs / Visualization have since removed a lot of this leg work:
// Here is a square – pseudo codey in the Pythonic/C/C++/C# mode.
Void square(x,y, xpos, ypos) {
Float x, xpos, y, ypos;
xbounds = x;
ybounds = y;
posx = xpos;
posy = ypos;
.
.
.
// draw the square with user specified color, position, and dimension, probably integrating OpenGL and other libraries.
}
In Objective-C, one would do much the same as any other C-based language, but it would resemble this after all of the instance variables and other set up is declared:
// Of course, various things have been left out for brevity sake for globals, but assuming you have all your instance variables and preparations in order, this will not be far off. Not how everything is bracketed. I hated this personally at first, but came to find it very useful as I went along. It helps keep things nice and organized. Also, whereas in other versions of C-type languages, you only have to write the code once and make calls – in Obj-C, frequently you have to do the following… every… single… time.
ViewDidLoad() {
CGRect square = CGRectMake(0.0f, 0.0f, 320.0f, 109.0f);
UIView *view = [[UIView alloc] initWithFrame:square];
view.backgroundColor = [UIColor redColor];
// If you like being self-referential: -- self.view = square; -- generally not recommended.
[self addSubview:view];
[view release];
}
As you noticed too, there is a release call. Everything that is ‘pointered’ and placed into the memory (has the little * in front of it for the non-programming savvy) must be released in the iOS environment, as there is no built-in garbage collection and memory leaks can begin to pile up very quickly. This is especially important in programming games; which tend to have a cascade of classes, local and global variables, and other issues that would make even a small handful of these catastrophic. While on a more powerful computer, things such as this might not be noticeable, we are working in a very constrained environment on the iPhone and iPad, given the built in hardware and will eventually cause the application to crash. It’s wonderfully powerful and versatile if you know what you are doing, but at the same time limited by the hardware. This forces the dev to become increasingly efficient as they become more experienced, due to the aforementioned constraints.
The aforementioned example may seem very simple, but there is actually a lot happening there and little details to keep track off. Forgetting a bracket or adding an extra will release a world of pain and frustration that can only be rivaled by forgetting a curly brace. Remember to follow the MVC and syntax standards!
The openness and versatility of the code comes with the cost that there are numerous pitfalls and deprecated methods that the unwary programmer can fall into that might not be readily apparent in the first few builds of the program, but if one runs into an incompatible or similarly named method or class call, trouble can arise very quickly. I learned this the hard way during some of my very first programs with Obj-C, where I might call for a timer of one call-type that may be completely incompatible with what the programmer and developer are trying dozens, if not hundreds of lines of code later. And it will not always catch as an exception or error within the compiler. Those errors are actually easy to deal with, and the internal compiler dump is pretty clear as to what the issue is. 90% of the time it will be an unused instance variable or mistyped call. Given all of that, the basic algorithms and other fun math features, variables, basic control referrals, and probabilities that come with writing games are fairly straightforward for those familiar with game programming, but one must always keep in line with Apple’s MVC or get b-slapped into debugging hell.
The dev might not even get a warning and will find that, they either have a program that does not work from the get go, or had worked previously but now either crashes when the new method/call/class/what have you is introduced, or behaves very oddly without any idea what is going on beyond a cryptic error message in the console, usually filled with hexadecimal code, various bits of assembly language, and even more archaic text regarding how a certain function is not recognized or that something that was previously linked now had changed without any information recognizable to the average hobbyist programmer like myself, and often results in consulting our best friend, Google or any number of more tech-savvy friends who might be available.
Then comes the decision on whether to program from a purely programmatic perspective or use the Interface Builder (IB) that comes with the programming suite XCode. For those who have been programming since the early days, doing things programmatically (and XCode comes loaded with convenience methods), this might be the most comfortable and most customizable way to do things. If you are a newer programmer and/or, like myself, are coming from a .NET or Visual IDE designer background, I’d recommend playing with IB, but be aware, especially when dealing with multi-tab programs to associate things with the proper view controllers. View Controllers and their importance are borne from the MVC standard that Apple has set. Fortunately, this is for our benefit, and one can quickly isolate problems within view controllers and their related classes, even if you are using only one ‘view’ in the entire subroutine. In an iOS program, all visual and interactive aspects come down to the various views. (UIImage, -Web, -Button, -Slider, etc.) The view controller helps further isolate and as the name suggests control and organize these various views into a manageable and legible format.
The nice thing about IB; despite its drawbacks (mostly requiring the dev to be extremely meticulous in checking links and class names), is that it is very much drag and drop and anyone who has worked in the .NET and Visual Studio framework will feel right at home after tinkering with it. For simpler programs, all the programmer has to do is generate the outlets and connect them. This is pretty intuitive once it gets done, but any change to classes, function calls, or overlapping outlets will cause things to get messy in short order, but this is true for any IDE. I prefer, at the current point in my career, actually doing it programmatically with @selectors in the interaction calls and defining variables and locations in the program itself rather than relying on Interface Builder due to its shortcomings, though it does work well for roughing the program out visually. This way, I can simply point at assets and outlets I have defined within the code rather than jumping back and forth between two programs that use entirely different parts of my brain and losing track of what I was doing.
Every time something is added or changed to the screen, a view is either added, changed, and redrawn, or removed. Going back to memory management, this can create a lot of issues if these are not disposed of properly. Having a View taking up memory even though it is not present, or has been removed from the visible interface will cause a very rapid and sometimes catastrophic memory leak, particularly if in that view had subviews where a lot was going on.
Thus why approved engines have become increasingly en vogue with game devs who have the capital to invest in the software. Others that are free likely exist but may not be as full featured or stable, which may result in an app getting rejected due to the standards of Apple, but when one is learning, that is really neither here nor there and Apple is quite helpful in helping you figure out what is wrong with your program. I have had a few demonstrated to me or researched and seen how they can circumvent a lot of the harder or more arcane legwork within the XCode/iOS environment.
Programs such as Angry Birds (Box2D, I believe), Epic Citadel (Unreal Engine Demo) and No! Human (Unity) were built in third-party engines that were/are approved by Apple for use in development of entertainment and gaming based apps. Other programmers and development houses opt to build their own engines within the COCOA/Obj-C framework and use that to power their programs while avoiding some of the nitty gritty. Still, it is my belief that it would behoove people who are new to iOS to actually sit down and try to write a game in Obj-C, as I did (a little word scramble game, mostly to play with the graphical capabilities), before diving into an engine and working from there. Though one can get away with doing C++-derived code mixed with Obj-C through an engine, there are certain neat features (accelerometers, gyroscope, etc) that may, as of this writing, may only be available and accessible through Obj-C in the iOS environment.
Fortunately for those of us who want to challenge ourselves and write a game primarily in the Obj-C language, XCode comes with a fairly robust debugging suite and SnapShot feature that allow developers to track their code line by line and back up to an earlier iteration if things simply become too hairy and frustrating. Unfortunately, debugging does not readily apply to IB as XCode and IB are technically separate programs and this is where many of the aforementioned unknowable errors can crop up. Much of the time, fixing these is a result of finding the misdirected link or incorrect ‘class identifier’ and switching it out until something works. After the first couple of times, this becomes second nature and fixed within minutes instead of hours. For those of us who either prefer programmatic approaches or do not want to deal with the IDE, we have the option to avoid it.
Being from a primarily visual background, programming is not the easiest thing for me to do, but it is something I enjoy and should I get a job in the gaming or animation industries, being familiar will likely help with building rapport with programmers and understanding their limitations. Furthermore, iOS is actually a great language for designers/artists, as it does have a robust and visually oriented bent – although asset management and proper coding can become an incredible chore, given that there are tedious and repetitive coding aspects to Obj-C. Cut and Paste will become one of your most used tools in Obj-C, like many other programming languages where you have to do the same thing repeatedly. Just be very careful with the syntax. Getting acquainted was a tough slog for me, personally, but once I did, I felt empowered and have in the future, whether I create games or other programs, an entirely new market opened up to me and as I build my repertoire, what will likely become an incredibly marketable skill. Obj-C has actually been around for quite awhile, but now is becoming a mature language with numerous books written specifically for the iOS environment and is rapidly building a vibrant programming community and a language I would highly recommend getting acquainted with, despite the aggravations it may cause in the beginning and will likely help make experienced programmers even more efficient.
Read more about:
Featured BlogsAbout the Author(s)
You May Also Like