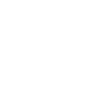
Featured Blog | This community-written post highlights the best of what the game industry has to offer. Read more like it on the Game Developer Blogs.
Chance, Probability, Luck, Percentage calculation for games.
When making games (and sometimes other software) where you need a chance of something either happening or not, it can get somewhat complicated to make it all work correctly.
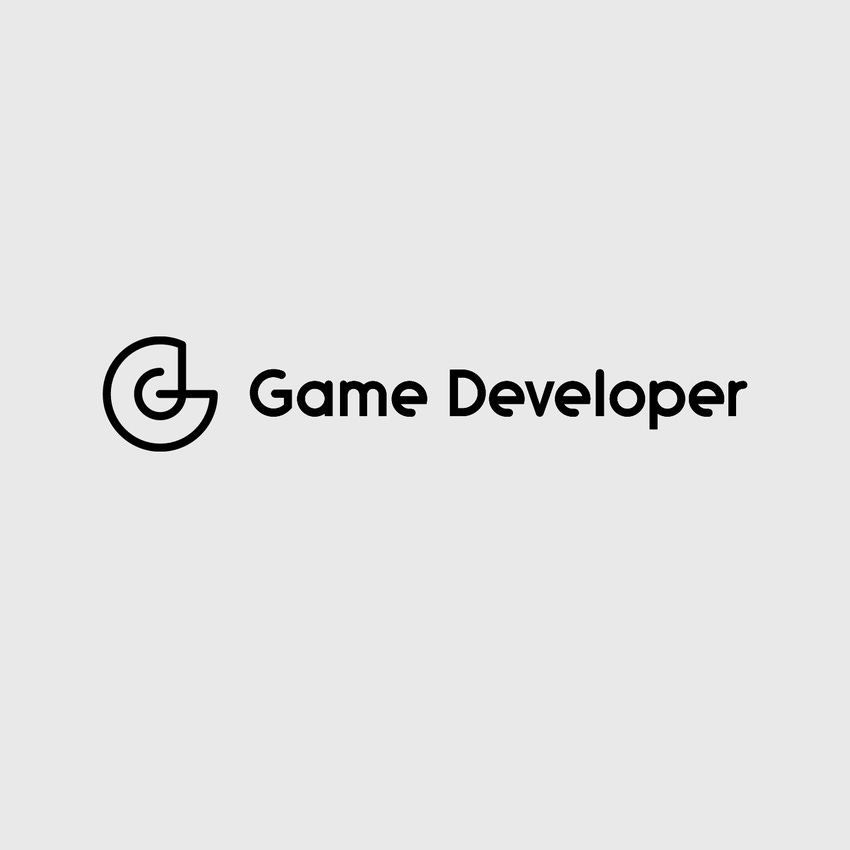
When making games (and sometimes other software) where you need a chance of something either happening or not, it can get somewhat complicated to make it all work correctly.
Roger Hågensen considers himself an Absurdist and a Mentat, hence believing in Absurdism and Logic. Has done volunteer support in Anarchy Online for Funcom. Voicework for Caravel Games. Been a Internet Radio DJ with GridStream. Currently works as a Freelancer, Windows applications programmer, web site development, making music, writing, and just about anything computer related really. Runs the website EmSai where he writes a Journal and publishes his music, ideas, concepts, source code and various other projects. Has currently released 3 music albums.
When do you need chance/probability in a game?
You may want a pure lucky chance, like say the player either hitting or not hitting something. A pure random pick of 0 or 1 (random number range pick of 0-1) will give around 50% chance and would work well in that case, and easy to implement.
But what about 90% chance of hitting, or 5% chance of hitting?
What if your game has modifiers like a bonus, or skill increase or items that increase the player chance of hitting, or maybe sometimes they may be hurt, thus reducing their chance of hitting, or a enemy cast negative buffs on the player.
Trying to create a random number range to handle all this is impossible (or is it?), and many end up making huge mistakes. Wrong calculations can lead to frustrated players when they "know" they should have hit based on the character attributes and skill,
yet they seem not to hit based on the percentage the game tells them they should have.
For example a hit chance of 99% should hit 99 out of 100 shots, but how many games can you remember where this has been true? And how many games have you noticed where 100% really doesn't match 100%?
The code!
The following code is in PureBasic a brilliant procedural basic based syntax, cheap license, and you can code for Windows, Mac and Linux. performance is similar to C and you can make tight standalone executables. For those with no familiarity with PureBasic I'm hoping that the syntax easy enough and suitable as pseudocode that you can port to your own preferred programming language.
The Chance_Luck() routine takes a percentage as chance/probability value.
Set it to 0 or lower and you will always get False back, set it to 100 or higher and you will always get True back.
Set it to 1-99 and you will get true returned based on the probability, so setting the value to 99 and then calling Chance_Luck() 100 times you should on average get true 99 times and false 1 time.
This is perfect for lucky chance checks where you might want to let the player either hit or miss, or get hit or missed but with a specific probability.
The Chance_Amount() routine takes a amount value and a percentage of chance/probability value.
Set the percentage to 0 or lower and you will always get amount of 0 back, set it to 100 or higher and you will always get the full amount back.
Set the percentage to 1-99 and you will get the amount back modified by the percentage probability, so setting the percentage to 99 and amount to 1000 then calling Chance_Amount() will return 901 to 1000 as the amount.
This is perfect for damage dealt or damage received checks where you might want the player to always deal at least 90%-100% damage for example.
;© Roger "Rescator" Hågensen, EmSai 2010. http://www.EmSai.net/
;Creative Commons Attribution License,
;http://creativecommons.org/licenses/by/3.0/
;Chance of success or failure given the percentage of probability.
;If 0% chance or lower (negative modifer overload, penality), failure always.
;If 100% chance or higher (positive modifer overload, bonus), success always.
;Otherwise 1% to 99% chance (+/- modifiers etc.).
Procedure.i Chance_Luck(percentage.i)
Protected result.i,chance.i=#False,range.i
If percentage>99
result=#True
ElseIf percentage>0 ;1% to 99% chance
range=100-percentage
chance=Random(99)+1 ;Kinda clunky but needed since the number range starts at 0 rather than 1.
If range
result=#True ;lucky chance
EndIf
EndIf
ProcedureReturn result
EndProcedure
;Chance of full amount given the percentage of probability.
;If 0% chance or lower (negative modifer overload, penality), amount is 0 always.
;If 100% chance or higher (positive modifer overload, bonus), amount is full always.
;Otherwise amount is 1% to 99% (+/- modifiers etc.).
Procedure.i Chance_Amount(amount.i,percentage.i)
Protected chance.f,result.i=#False,range.i
If percentage>99
result=amount
ElseIf percentage>0
range=100-percentage ;Get range based on percentage
chance=Random(range-1)+1 ;Kinda clunky but needed since the number range starts at 0 rather than 1.
chance=(chance+percentage)/100.0 ;Create the modifier based on percentage
result=amount*chance ;Apply modifer
EndIf
ProcedureReturn result
EndProcedure
Define.i i,n,s,percentage
;Lucky chance test
n=100000
percentage=50
DisableDebugger
For i=1 To n
s+Chance_Luck(percentage)
Next
EnableDebugger
Debug StrD(((s/n)*100.0))+"% lucky chance average with "+Str(percentage)+"% probability."
;Amount probability test
n=1000
percentage=90
Debug Str(Chance_Amount(n,percentage))+" of max amount "+Str(n)+" with "+Str(percentage)+"% probability."
Chance vs Probability!
The routines are very simple, so what is the point of this being a Tips really?
The reason is that many confuses the idea of Chance and Probability, as chance always have a 50/50 chance of happening or not happening. While probability is the likelihood of it happening or not. In other words probability is chance+bias.
So think about impossible as a synonym for improbable, and possible as a synonym for probable. You may think it is impossible for humans to fly on their own, but its' not, it's simply highly improbable we'll be able to ever. You may think eating will always be possible, but you could be wrong, we could evolve to not need to eat, eating is simply highly probably to remain a human necessity.
The illusionist Derren Brown once tossed ten coins all heads in a row, the probability of that is very low, how did he do it?
He and the TV crew spent over 9 hours, where he again and again started counting from 1, until 9 hours later he finally managed ten heads in a row.
Conclusion!
So the next time you think that there is a probability of something happening 90% of the time, does not mean it actually will. There is a likelihood it will, but no guarantee.
But with the two routines above, you will be guaranteed to have the bias needed to make the chance match the expected perceived probability.
Those playing your game will have no idea how, but they will feel that the game is fair.
As it is the perceived probability that people expect, rather than what chance actually is, pure luck.
Read more about:
Featured BlogsAbout the Author(s)
You May Also Like