Unity UI Button Tip - Changing The Text Label Via Script
With the new Unity UI, working with text labels on buttons can be tedious since you need to deal with two separate GameObjects. We can simplify this with a little code.
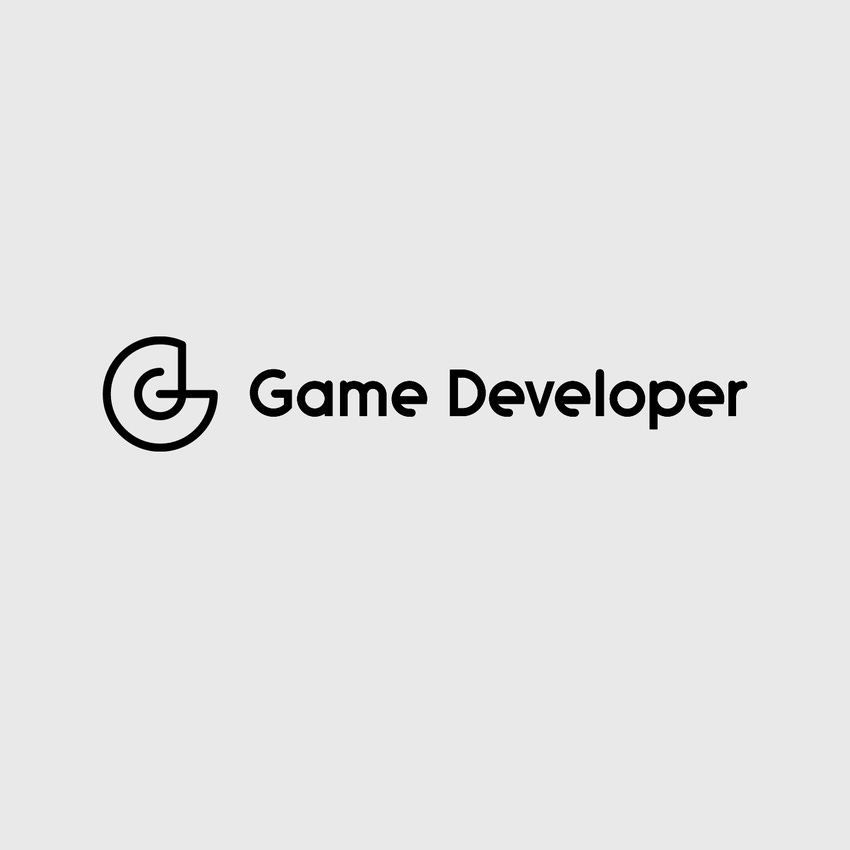
While working on a project using Unity's new UI, I adopted some conventions around using the Button element that I think other developers would find useful. In particular, I'd like to talk about how to easily access the text labels of buttons. If you have experience with the Immediate Mode GUI, you will find that the way it's done with the new UI is different. This is my attempt at simplifying the process.
Note that the UI Button prefab is actually made up of two distinct GameObjects - the actual Button element, and a UI Text element as its child. To access both the button and the text, you'll have to place each of them in separate variables:
public Button myButton; public Text myButtonText;
While this is generally not a problem, it can be tedious once it's time to assign instances to these variables via the inspector. For each button, you'll have to make assignments twice - one for the button itself, and another for the text element. This is prone to error - it is possible to miss assigning one of the two, or to assign non-matching Button and Text GameObjects to the variables.
To fix this, we can write a little code that will allow us to access both the Button and the Text elements through a single MonoBehaviour. Here goes:
public class ButtonAndText : MonoBehaviour { public Button button; public Text text; void Awake () { button = GetComponent<Button> (); foreach (Transform t in transform) { // might not be obvious text = t.GetComponent<Text> (); } } }
A simple solution to a simple problem! Most of this is straightforward - create a new MonoBehaviour that aggregates the Button and Text components. But what might not be obvious here is how we get to the Text element. We need to note that:
The Text GameObject is the only child of the Button GameObject, and...
So by placing this MonoBehaviour on our Button GameObject, we can automatically obtain references to Button/Text pairs while minimizing the risk for human error and decreasing the manual work we have to do with assignments. To easily use this in your project, instantiate a default Button object, add the ButtonAndText component to it, then keep the result as a prefab. Use this prefab whenever you need a button. And in your code, you can refer to buttons like this:
public ButtonAndText myButton;
And manipulate them like this:
myButton.button.interactable = false; myButton.text.text = "Hello!";
With a little code, we are able to manipulate buttons and their corresponding text labels with less work.
Download the source code
The project folder for the ButtonAndText component is available for download as part of a grab bag of code samples that I've released previously (started out with Unity networking samples but now being updated to include other stuff). Included in the project folder is an example scene that demonstrates the ButtonAndText component together with a couple more button-related examples (clicking and holding) which I'll write articles for soon. Also included in the project folder are button prefabs that are ready to use for your own projects, so be sure to grab the source code download if you're interested in those.
Read more about:
BlogsAbout the Author(s)
You May Also Like