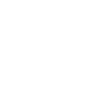
Featured Blog | This community-written post highlights the best of what the game industry has to offer. Read more like it on the Game Developer Blogs.
Two Unity Things
A post in which I introduce the first few lines of code I ever wrote while developing stuff in Unity3D and hope that it paves the way for further, better, posts!
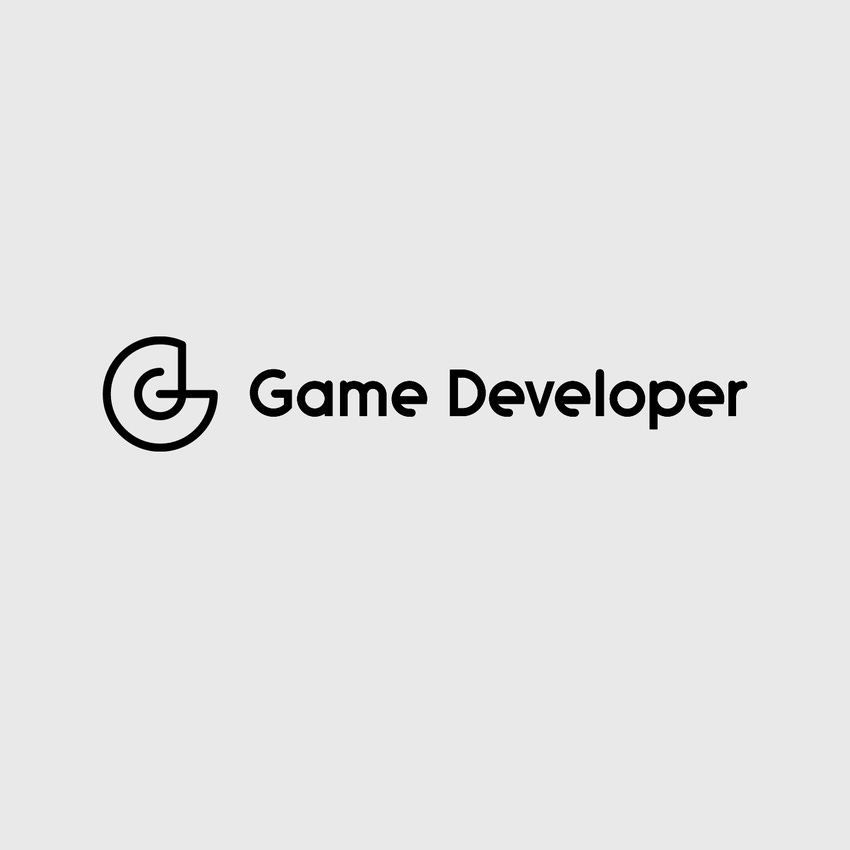
Ok, not the best title for my first blog post on Gama but I figured starting small would be a good introduction to my writing style (if anyone is reading this of course). So, Unity3D is, as implied by its name, a pretty good 3D game development framework. It's a shame then that I use it mainly for developing 2D games. Oh well... I guess that's a topic for a later post.
After a week or so with the engine I started noticing two things. First, I really really wanted an easy way to reset a group of game-objects to zero. And secondly, I found that creating instances of GameObject in code can be repetetive over my particular needs from them. So without further ado let me introduce answer number one: Resetting transformations using a menu item and a shortcut key (or hotkey or however you want to call these things).
The code itself is not complicated;
public static class ResetSelectedTransforms
{
[MenuItem("Utilities/Reset Selected Transforms &z")]
public static void Perform()
{
foreach (var selected in Selection.gameObjects)
{
var transform = selected.transform;
transform.localPosition = Vector3.zero;
transform.localRotation = Quaternion.identity;
transform.localScale = Vector3.one;
}
}
}
Notice that I added the '&z' character to the menu item's name; this causes Unity to associate the 'alt+z' shortcut to our menu and makes it easier to call it (remember: lazy is good!). And finally see that we're resetting only the local properties of the transform, this way we're avoiding a host of issues concerning the parenting hierarchy of the object.
Using this menu item a few times and writing more code for the game I also found that resetting transforms is something I need to do sometimes during Unity's runtime environment (rather than just while editing - more on my building process and separation of projects in a later post). So let's extract this into a useful utility/extension method.
public static class TransformResetExtensions
{
public static void ResetLocal(this Transform transform)
{
transform.localPosition = Vector3.zero;
transform.localRotation = Quaternion.identity;
transform.localScale = Vector3.one;
}
public static void ResetGlobal(this Transform transform)
{
transform.position = Vector3.zero;
transform.rotation = Quaternion.identity;
// Unity hasn't exposed a setting on the lossy scale;
}
}
Next, and last, item on the agenda for today; wrapping the GameObject creation in a useful factory method.
One of the main reasons for creating this wrapper is to allow easy parenting. Now, code!
public static class NewGameObject
{
public static GameObject Make(string name, GameObject parent)
{
return Make(name, parent.transform);
}
public static GameObject Make(string name, Component parent = null)
{
var new_entity = new GameObject(name);
new_entity.transform.parent = GetAsParentTransform(parent);
new_entity.transform.ResetLocal();
new_entity.AddComponent();
return new_entity;
}
private static Transform GetAsParentTransform(Component component)
{
if (component == null)
return null;
if (component is Transform)
return component as Transform;
return component.transform;
}
}
Again, nothing overly complicated going on there but I will explain a few points. First of all, the GetAsParentTransform() method. Because the code allows parenting new instances to any component (or game object) we need to check whether that particular component is a transform (and then use it as the parent) or whether it's any other component (and then use its transform component as the parent).
The second point you (yes YOU) must have noticed is that we're adding something called a TransformLock. That's my own special sauce cooked up for Unity and, like all other things, will be the topic of another post.
Alright, until next time - so long and thanks for all the fish; also, go check out our awesome kickstarter for Buck.
"Truth is I haven't played much since the baby came in June, but give me half a minute and I'll get this fiddle back in tune."
Read more about:
Featured BlogsAbout the Author(s)
You May Also Like