Sponsored By
Rotate, zoom and move your camera with your mouse in unity3d
I've been working on a new project, which will use mouse to move and rotate your camera. Here is the code!
2 Min Read
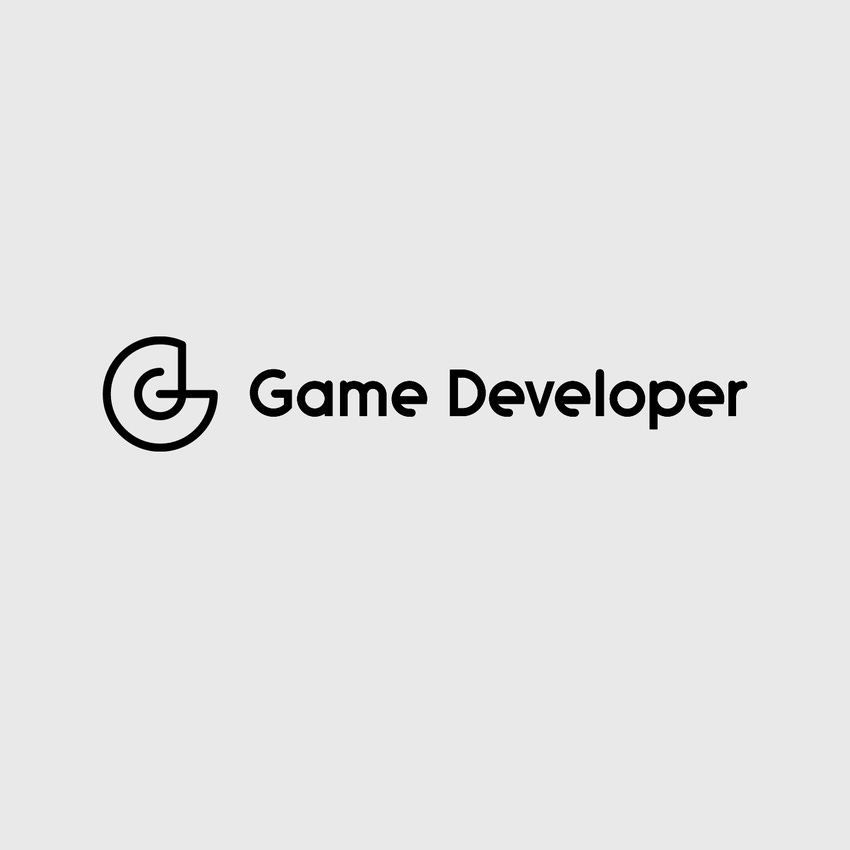
I've been working on a new project, which will use mouse to move, zoom and rotate your camera. I've been searching on internet but never find a good solution. But I figured it out, here is the code.
To use the code, create a new c# script, attach it to a camera, then remove start and update function, then paste them in.
public enum RotationAxes { MouseXAndY = 0, MouseX = 1, MouseY = 2 } public RotationAxes axes = RotationAxes.MouseXAndY; public float sensitivityX = 2F; public float sensitivityY = 2F; public float minimumX = -360F; public float maximumX = 360F; public float minimumY = -90F; public float maximumY = 90F; float rotationY = -60F; // For camera movement float CameraPanningSpeed = 10.0f; void Update() { MouseInput(); } void MouseInput() { if (EventSystem.current.IsPointerOverGameObject()) { return; } if (Input.GetMouseButton(0)) { } else if (Input.GetMouseButton(1)) { MouseRightClick(); } else if (Input.GetMouseButton(2)) { MouseMiddleButtonClicked(); } else if (Input.GetMouseButtonUp(1)) { ShowAndUnlockCursor(); } else if (Input.GetMouseButtonUp(2)) { ShowAndUnlockCursor(); } else { MouseWheeling(); } } void ShowAndUnlockCursor() { Cursor.lockState = CursorLockMode.None; Cursor.visible = true; } void HideAndLockCursor() { Cursor.lockState = CursorLockMode.Locked; Cursor.visible = false; } void MouseMiddleButtonClicked() { HideAndLockCursor(); Vector3 NewPosition = new Vector3(Input.GetAxis("Mouse X"), 0, Input.GetAxis("Mouse Y")); Vector3 pos = transform.position; if (NewPosition.x > 0.0f) { pos += transform.right; } else if (NewPosition.x < 0.0f) { pos -= transform.right; } if (NewPosition.z > 0.0f) { pos += transform.forward; } if (NewPosition.z < 0.0f) { pos -= transform.forward; } pos.y = transform.position.y; transform.position = pos; } void MouseRightClick() { HideAndLockCursor(); if (axes == RotationAxes.MouseXAndY) { float rotationX = transform.localEulerAngles.y + Input.GetAxis("Mouse X") * sensitivityX; rotationY += Input.GetAxis("Mouse Y") * sensitivityY; rotationY = Mathf.Clamp(rotationY, minimumY, maximumY); transform.localEulerAngles = new Vector3(-rotationY, rotationX, 0); } else if (axes == RotationAxes.MouseX) { transform.Rotate(0, Input.GetAxis("Mouse X") * sensitivityX, 0); } else { rotationY += Input.GetAxis("Mouse Y") * sensitivityY; rotationY = Mathf.Clamp(rotationY, minimumY, maximumY); transform.localEulerAngles = new Vector3(-rotationY, transform.localEulerAngles.y, 0); } } void MouseWheeling() { Vector3 pos = transform.position; if (Input.GetAxis("Mouse ScrollWheel") < 0) { pos = pos - transform.forward; transform.position = pos; } if (Input.GetAxis("Mouse ScrollWheel") > 0) { pos = pos + transform.forward; transform.position = pos; } }
Read more about:
BlogsAbout the Author(s)
Daily news, dev blogs, and stories from Game Developer straight to your inbox
You May Also Like