Learning Gideros in 10 easy steps
This article explains 10 simple snippets on how to achieve 10 simple tasks in Gideros using Lua, to get you started on developing cross platform mobile games with Gideros
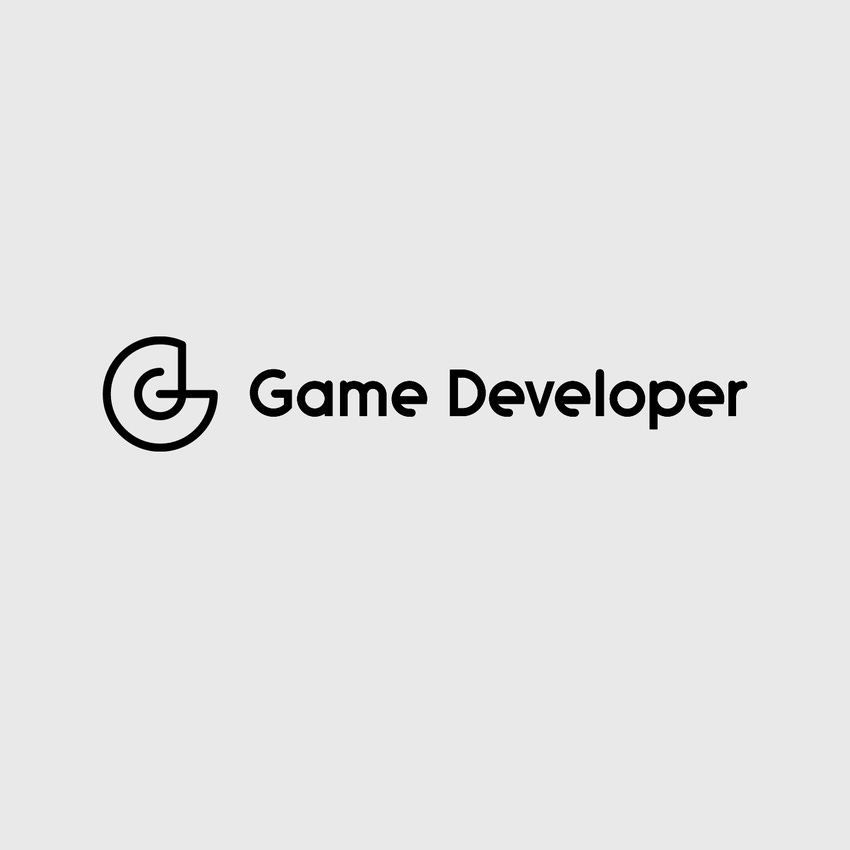
With so many options of cross platform tools to develop on multiple platforms, it is getting quite hard to decide on which tool is better to use.
When evaluating them, you can usually check the feature list, supported platforms and prices, all the information that can help you decide. But what you usually can't evaluate from the first sight, is the learning curve and the feeling of the tool while developing/managing project
That's why I've prepared 10 simple steps you can take while trying to learn more about Gideros and evaluate it as your choice for cross platform mobile development tool
1. Display image
To display image, we firstly create the Texture object by providing path to the image file and optional boolean parameter which indicates if the image should be filtere (anti-alised)
Then we create Bitmap object, position it at some coordinate (default are 0,0) and add it to the stage to be rendered
local bmp = Bitmap.new(Texture.new("images/ball.png", true)) bmp:setPosition(100, 100) stage:addChild(bmp)
2. Display text
To display text, we firstly need to create Font object, in this case we will use TTFont. We provide path to the font file, size of the text and optional boolean parameter which indicates if the image should be filtere (anti-alised)
Then we create TextField object by passing Font object and text that we want to display.
After that we simply set position of the text and add it to the stage to be rendered
local tahomaFont = TTFont.new("fonts/tahoma.ttf", 20, true) local text = TextField.new(tahomaFont, "Hello World!!!") text:setPosition(100, 100) stage:addChild(text)
3. Draw shape
We can also draw arbitrary shapes
To accomplish that, we need to create Shape object and set its fill and line styles.
We will use a solid red color the fill style and 5px width blue line with 1 alpha (or full opacity)
Then we can begin drawing arbitrary shape and once we've done, we simply set position of the text and add it to the stage to be rendered
local shape = Shape.new() shape:setFillStyle(Shape.SOLID, 0xff0000) shape:setLineStyle(5, 0x0000ff, 1) shape:beginPath() shape:moveTo(0,0) shape:lineTo(0, 100) shape:lineTo(100, 100) shape:lineTo(100, 0) shape:lineTo(0, 0) shape:endPath() shape:setPosition(200, 100) stage:addChild(shape)
4. Groups or layers
We can use Sprite objects to group other objects (or separate them in different layers) as images, texts and shapes
To do that, we simply create Sprite object and add other objects as its child
After that we can manipulate the whole group easily, as for example, changing position of all element by simply changing position of parent element.
And of course as usually, we add it to the stage to be rendered
local sprite = Sprite.new() sprite:addChild(bmp) sprite:addChild(text) sprite:addChild(shape) sprite:setPosition(100, 100) stage:addChild(sprite)
5. Playing sounds
To play sounds, we simply need to create the Sound object, by providing path to mp3 or wav file, which we are going to play
And then call play to play it, which will create a channel currently playing
We can then stop the channel any time we want or let the sound finish
local sound = Sound.new("music.mp3") local channel = sound:play() -- after some time -- channel:stop()
6. Animating transformations
We can animate any transformation, as scaling, position or even rotation of the objects
So here we create a Bitmap object, and set it's anchor point to 0.5, 0.5, which will reference the center of the object (so it would rotate around the center and not top left corner).
Then we set up an enter frame event, which is executed on every frame, and change the rotation of image by 5 degrees on each frame
local bmp = Bitmap.new(Texture.new("ball.png", true)) bmp:setAnchorPoint(0.5, 0.5) bmp:setPosition(100, 100) stage:addChild(bmp) stage:addEventListener(Event.ENTER_FRAME, function() bmp:setRotation(bmp:getRotation()+5) end)
7. Frame animations
To create frame animation, we firstly need to load each separate frame, either from TexturePack or simply images as Bitmap objects.
Then we create a MovieClip object and pass table with arranged frames and frame intervals to it (quite similarly as timeline in Action Script)
Then we loo[ the animation by setting goto action and start playing.
And the last thing as previously, we set its position and add it to the stage to be rendered
--load frames local frames = {} local bmp for i = 1, 9 do bmp = Bitmap.new(Texture.new("ball"..i..".png", true)) bmp:setAnchorPoint(0.5, 0.5) frames[#frames+1] = bmp end --arrange frames local ballAnimation = MovieClip.new{ {1, 5, frame[1]}, {6, 10, frame[2]}, {11, 15, frame[3]}, {16, 20, frame[4]}, {21, 30, frame[5]}, {31, 40, frame[6]}, {41, 45, frame[7]}, {46, 50, frame[6]}, {51, 55, frame[8]}, {56, 60, frame[6]}, {61, 65, frame[7]}, {66, 70, frame[6]}, {71, 75, frame[8]}, {76, 80, frame[6]}, {81, 85, frame[7]}, {86, 90, frame[6]}, {91, 95, frame[8]}, {96, 100, frame[5]}, {101, 110, frame[4]}, {111, 115, frame[3]}, {116, 120, frame[2]}, {121, 125, frame[1]}, {126, 200, frame[2]}, } --loop animation ballAnimation:setGotoAction(200, 1) --start playing ballAnimation:gotoAndPlay(1) ballAnimation:setPosition(160, 240) stage:addChild(ballAnimation)
8. Detecting click events
We will add additional methods to created Bitmap object which would scale it up on mouse down and scale back on mouse up
Inside these events we will check if the object was clicked, using hitTestPoint method
Then we simply attach these event listener to corresponding events
local bmp = Bitmap.new(Texture.new("ball.png", true)) bmp:setAnchorPoint(0.5, 0.5) bmp:setPosition(100, 100) stage:addChild(bmp) function bmp:onClick(e) if self:hitTestPoint(e.x, e.y) then self:setScale(1.5) end end function bmp:onRelease(e) if self:hitTestPoint(e.x, e.y) then self:setScale(1) end end bmp:addEventListener(Event.MOUSE_DOWN, bmp.onClick, bmp) bmp:addEventListener(Event.MOUSE_UP, bmp.onRelease, bmp)
9. Getting user input
We can also get user input from TextInputDialog, for example, if we need a user to provide username.
We cretae a onComplete event handler, to check if user did not cancel the dialog and retrieve entered text
local username = "Player1" local textInputDialog = TextInputDialog.new("Change username", "Enter yout user name", username, "Cancel", "Save") local function onComplete(e) if e.buttonIndex then username = e.text end end textInputDialog:addEventListener(Event.COMPLETE, onComplete) textInputDialog:show()
10. Save/read data persistently
Sometimes in games we also need to save data persistently
For that we can create to function, to serialize any table data using json and save it by key
Then we create a second function to retrieve the save information by the same provided key
require "json" function saveData(key, value) local contents = json.encode(value) --create file local file = io.open( "|D|"..key, "w" ) --save json string in file file:write( contents ) --close file io.close( file ) end function getData(key) local value local file = io.open( "|D|"..key, "r" ) if file then --read contents local contents = file:read( "*a" ) --decode json value = json.decode(contents) --close file io.close( file ) end return value end --try to read information local someData = getData("someData") -- if no information, create it if not someData then someData = {"some text", 42} --save data saveData("someData", someData) print("Creating someData") else print("Read someData", someData[1], someData[2]) end
Further reading
Of course there are much more to Gideros than this. This article only shows some code snippets to accomplish simple tasks, but Gideros offers much more, starting from killer features like 1 click on device testing, automatic scaling modes and choosing image resolution automatically, to texture packs, OOP system and physics.
If you are interested to learn more about these Gideros features, check out Gideros Mobile Game Development book by Arturs Sosins, published by Packt publishing.
Read more about:
BlogsAbout the Author(s)
You May Also Like